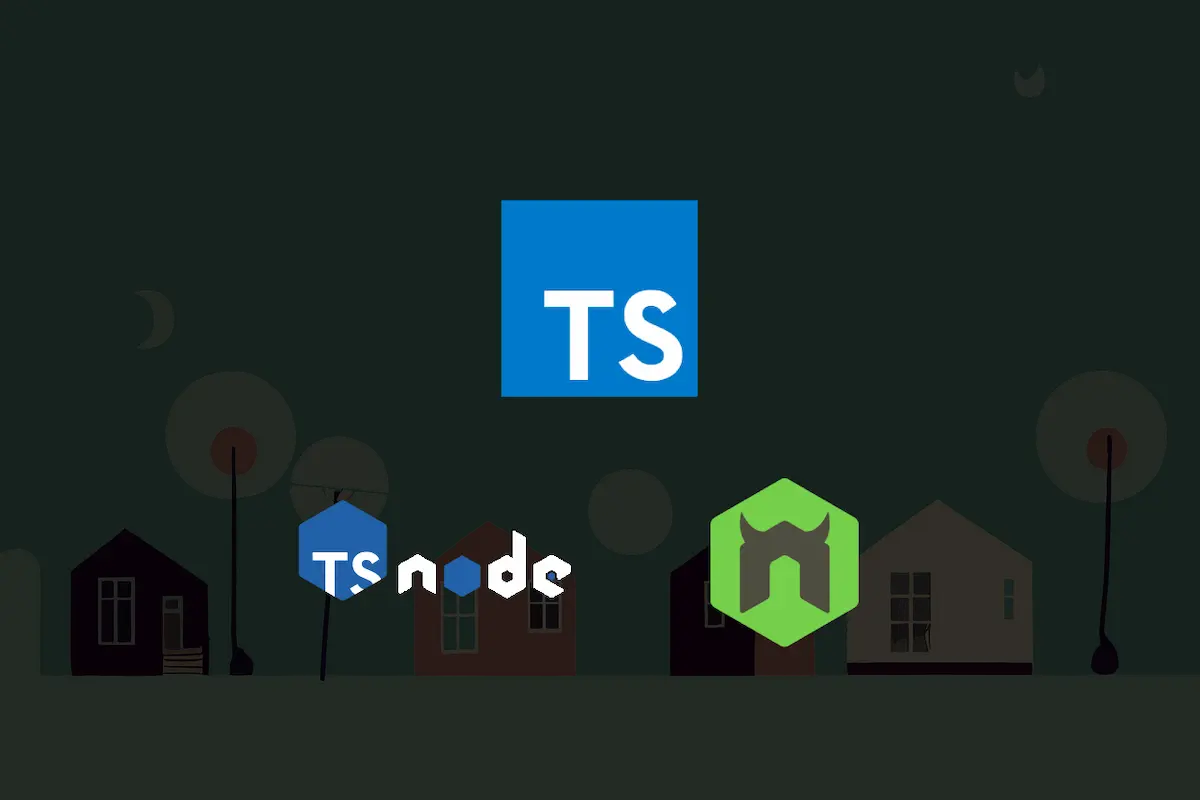
Run TypeScript Without Compiling
- Mohammad Abu Mattar
- Programming
- 02 Dec, 2022
- 02 Mins read
Introduction
In this post, I will show you how to run TypeScript without compiling it to JavaScript. This is useful for debugging and testing. In this post, I will show you how to do it.
Setup a TypeScript Project
Step 1: Create a Directory
Create a directory for the project.
# Create a directorymkdir run-typescript-without-compiling
# Change directorycd run-typescript-without-compiling
Step 2: Initialize a Node.js Project
Initialize a Node.js project.
# Initialize a Node.js projectyarn init -y
Step 3: Install TypeScript and @types/node
Install TypeScript and @types/node
.
# Install TypeScript and @types/nodeyarn add -D typescript @types/node
Step 4: Initialize a TypeScript Project
Initialize a TypeScript project.
# Initialize a TypeScript projectyarn tsc --init
Step 5: Create a TypeScript File
Create a TypeScript file.
# Create a src directorymkdir src
# Create a TypeScript filetouch src/index.ts
Step 6: Example Code
Add the following code to the TypeScript file.
1const sum = (a: number, b: number): number => a + b;2const subtract = (a: number, b: number): number => a - b;3const multiply = (a: number, b: number): number => a * b;4const divide = (a: number, b: number): number => a / b;5
6console.log(sum(1, 2));7console.log(subtract(1, 2));8console.log(multiply(1, 2));9console.log(divide(1, 2));
Step 7: Run the TypeScript File
Now, we will install ts-node
and run the TypeScript file.
# Install ts-nodeyarn add -D ts-node
Add the following code to the package.json
file.
1{2 ...3 "scripts": {4 "playground": "ts-node src/index.ts",5 "playground:watch": "nodemon -e ts -w . -x esr src/index.ts",6 "build": "tsc",7 "build:watch": "tsc -w",8 "build:debug": "tsc --sourceMap",9 "build:debug:watch": "tsc -w --sourceMap",10 "start": "node dist/index.js",11 "start:watch": "nodemon -e js -w dist -x esr dist/index.js"12 },13 ...14}
NOTE:
nodemon
is a utility that will monitor for any changes in your source and automatically restart your server. You can install it usingyarn global add nodemon
ornpm install -g nodemon
.
Run the TypeScript file.
# Run the TypeScript fileyarn playground
# Output3-120.5
Conclusion
I demonstrated how to run TypeScript in this article without converting it to JavaScript. For testing and troubleshooting, this is helpful. I demonstrate how to accomplish that in this post.