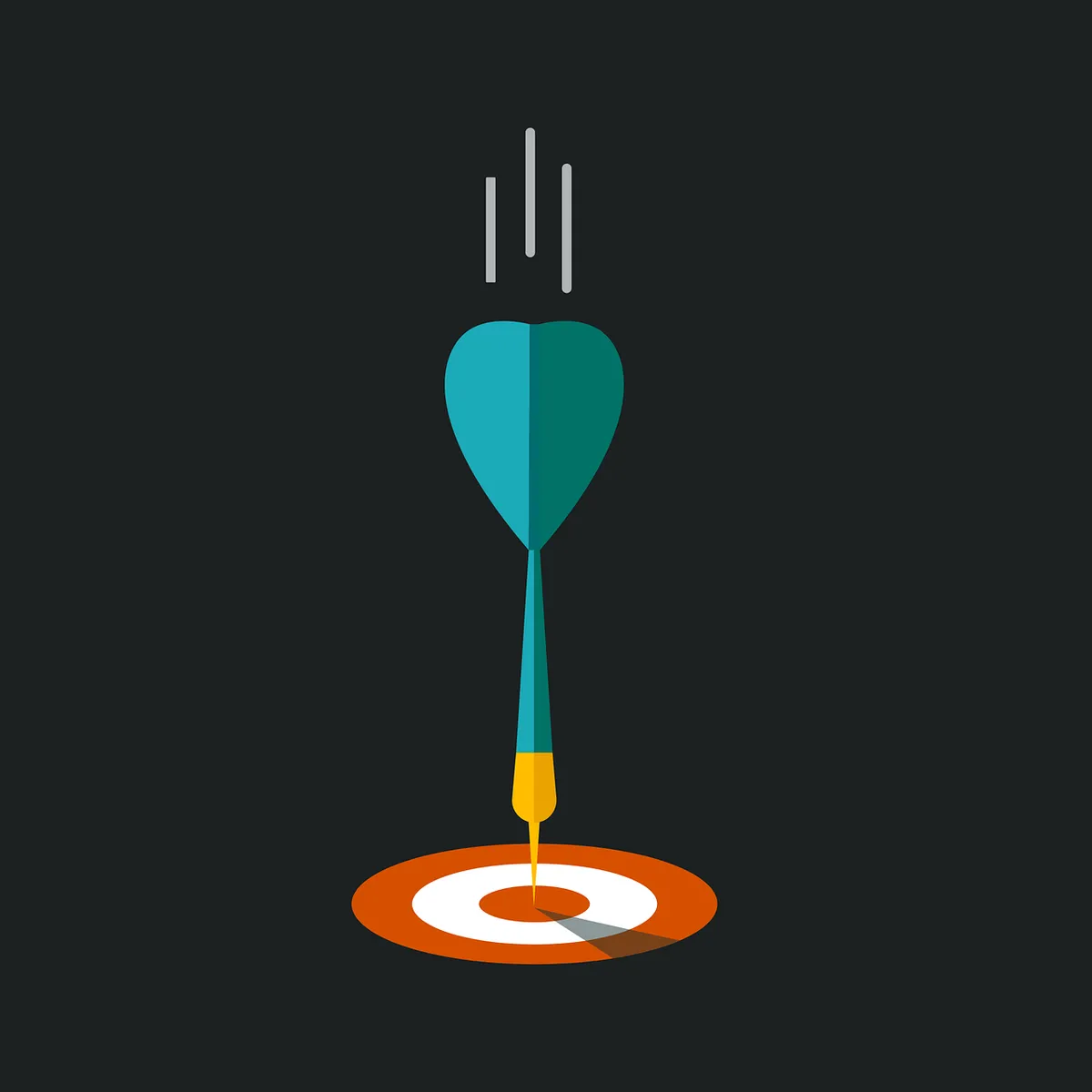
The ORM Dilemma: To Use or Not to Use
- Mohammad Abu Mattar
- Programming , Backend
- 24 Aug, 2023
- 06 Mins read
Introduction
In the world of software engineering, seasoned professionals are often confronted with pivotal decisions that wield substantial influence over the course and outcome of a project. Among these choices is the perennial dilemma of whether to employ an Object-Relational Mapping (ORM) tool for handling database interactions. Should you eschew an ORM entirely? Or should you harness the convenience it offers when working with technologies like Node.js and TypeScript, particularly in conjunction with Express? This article endeavors to dissect the pros and cons of employing an ORM and provide insights into scenarios where alternatives might be a more judicious choice.
A Glimpse into ORM
Before we plunge into the nuanced discourse of whether or not to utilize ORM, let’s briefly acquaint ourselves with what ORM entails and the functions it serves. At its core, an Object-Relational Mapping tool is a software framework that streamlines communication between an application and a relational database. It adroitly abstracts the intricacies of database interactions, enabling developers to interact with database entities as if they were conventional objects in their chosen programming language.
The Advantages of ORM
ORM proffers several compelling advantages that render it an alluring choice for developers:
-
Database Complexity Abstraction: One of ORM’s prime virtues lies in its ability to shield developers from the labyrinthine depths of SQL and the idiosyncrasies of database-specific operations. This abstraction proves invaluable, particularly when handling intricate queries.
-
Seamless Language Integration: ORM seamlessly dovetails with the programming language being employed. This harmonious integration permits developers to manipulate database records using native language constructs, culminating in code that is not only more maintainable but also notably more readable.
-
Cross-Database Compatibility: A plethora of ORM libraries offer robust support for multiple database systems, making the task of transitioning from one database to another a relatively painless endeavor. This flexibility can prove invaluable in evolving projects.
-
Expedited Development: ORM expedites the development process by alleviating the burden of crafting boilerplate code for routine database operations such as Create, Read, Update, and Delete (CRUD) actions.
While the advantages of ORM are compelling, we must now pivot our attention to the flip side and meticulously examine the disadvantages.
The Disadvantages of ORM
Undoubtedly, ORM is a potent tool, but it does not traverse the software landscape unburdened. Here are the principal disadvantages that warrant careful consideration:
1. Performance Overheads
Introducing ORM into the mix invariably adds an abstraction layer between your application and the database. While this abstraction might be conducive to code readability and maintainability, it often exacts a performance toll. The SQL queries generated by ORM may not always be as optimized as handcrafted SQL, particularly when grappling with intricate queries or high-throughput scenarios.
2. The Learning Curve
The adoption of an ORM tool often entails navigating a learning curve. Developers must acquaint themselves with the intricacies of the ORM’s Application Programming Interface (API), a process that can consume valuable time. Additionally, comprehending how the ORM translates high-level operations into SQL queries becomes paramount when striving to optimize performance.
3. Limited Control
ORM’s modus operandi involves abstracting database operations, which inherently implies a degree of control relinquishment over the SQL queries it generates. In scenarios necessitating fine-grained control over queries for performance optimization, the rigid structure of ORM might prove restrictive.
4. Code Bloat
While ORM effectively trims the fat of boilerplate code in common scenarios, it can paradoxically lead to code bloat in more complex situations. Achieving fine-grained control over database interactions often mandates the crafting of custom code within the confines of the ORM framework, a practice that can be verbose and challenging to maintain.
The Necessity of ORM: A Delicate Balance
Having scrutinized both the advantages and disadvantages of ORM, a pertinent question lingers: Is an ORM an indispensable tool? The resounding answer is, “It depends.”
When to Contemplate the Adoption of ORM
-
Rapid Prototyping: If your project’s primary objective revolves around expeditiously materializing a minimum viable product (MVP), an ORM can serve as an invaluable ally. It liberates you from the quagmire of SQL queries, allowing you to channel your energies into molding the logic of your application.
-
Team Expertise: If your development ensemble boasts greater proficiency in the programming language of your choice as opposed to SQL, then opting for ORM is a judicious course of action. It empowers your team to function efficaciously, culminating in the delivery of high-quality code.
-
Cross-Database Compatibility: Should your project necessitate support for multiple database systems, an ORM shines as a pragmatic choice. It transcends the discrepancies that segregate databases, rendering transitions from one database to another a relatively seamless endeavor.
When to Exercise Caution with ORM
-
Exacting Performance Demands: In scenarios where your application is subjected to stringent performance prerequisites, particularly when grappling with intricate queries or high transaction rates, the deployment of raw SQL or a database-specific library might be the wiser selection. This affords you the latitude to fine-tune SQL queries for optimal performance.
-
Database-Specific Features: Projects that heavily rely on database-specific features or the execution of advanced SQL operations that elude the grasp of ORM might discover greater efficiency in resorting to native SQL.
-
Requisite Query Control: Should the exigencies of your project necessitate granular control over the SQL queries executed by your application, the use of ORM may impose unwarranted limitations. In such instances, the composition of custom SQL queries emerges as the more judicious course of action.
Pragmatic Alternatives to ORM
For scenarios where the adoption of ORM might not align seamlessly with your project’s requisites, it becomes imperative to explore pragmatic alternatives. Below, we outline a couple of alternatives worthy of contemplation.
1. Query Builders
Query builders, exemplified by libraries such as Knex.js, proffer a middle-ground solution. They empower you to programmatically construct SQL queries using JavaScript, striking a harmonious balance between raw SQL and the abstraction provided by ORM. Query builders become especially invaluable when the need for query control converges with the desire for code that remains readable.
Consider a TypeScript and PostgreSQL example using Knex.js:
import * as Knex from 'knex';
const knex = Knex({ client: 'pg', connection: { host: 'your-database-host', user: 'your-username', password: 'your-password', database: 'your-database-name', },});
async function getUsers() { return await knex.select('*').from('users');}
async function addUser(user: any) { return await knex('users').insert(user);}
async function getComplexData( country: string, orderDate: Date, category: string,) { // Define Common Table Expressions (CTEs) const usersFromCountry = knex('users').where('country', country); const ordersFromLast30Days = knex('orders').where( 'order_date', '>=', orderDate, ); const booksOrderItems = knex('order_items') .join('products', 'order_items.product_id', '=', 'products.id') .where('products.category', category);
// Build the main query using CTEs const query = knex .with('users_from_country', usersFromCountry) .with('orders_from_last_30_days', ordersFromLast30Days) .with('books_order_items', booksOrderItems) .select( 'users_from_country.name', 'orders_from_last_30_days.order_date', 'books_order_items.product_name', ) .from('users_from_country') .leftJoin( 'orders_from_last_30_days', 'users_from_country.id', 'orders_from_last_30_days.user_id', ) .leftJoin( 'books_order_items', 'orders_from_last_30_days.id', 'books_order_items.order_id', );
return query;}
2. Raw SQL
In cases where optimal performance is paramount, and you yearn for absolute control over your queries, the embrace of raw SQL queries emerges as the superior choice. While this path demands a heightened level of diligence to fend off the specter of SQL injection, it confers an unparalleled degree of control and efficiency.
Here’s an example of TypeScript code executing a raw SQL query with the pg
library for PostgreSQL:
import {Pool} from 'pg';
const pool = new Pool({ user: 'your-username', host: 'your-database-host', database: 'your-database-name', password: 'your-password', port: 5432, // PostgreSQL default port});
async function getUsers() { const client = await pool.connect(); try { const result = await client.query('SELECT * FROM users'); return result.rows; } finally { client.release(); }}
async function addUser(user: any) { const client = await pool.connect(); try { const query = { text: 'INSERT INTO users(name, email) VALUES($1, $2)', values: [user.name, user.email], }; await client.query(query); } finally { client.release(); }}
async function getComplexData( country: string, orderDate: Date, category: string,) { const client = await pool.connect();
try { // Define the SQL query with placeholders for parameters const sqlQuery = ` WITH users_from_country AS ( SELECT * FROM users WHERE country = $1 ), orders_from_last_30_days AS ( SELECT * FROM orders WHERE order_date >= $2 ), books_order_items AS ( SELECT * FROM order_items JOIN products ON order_items.product_id = products.id WHERE products.category = $3 )
SELECT users_from_country.name, orders_from_last_30_days.order_date, books_order_items.product_name FROM users_from_country LEFT JOIN orders_from_last_30_days ON users_from_country.id = orders_from_last_30_days.user_id LEFT JOIN books_order_items ON orders_from_last_30_days.id = books_order_items.order_id `;
// Execute the SQL query with parameters const result = await client.query(sqlQuery, [country, orderDate, category]); return result.rows; } finally { client.release(); }}
Conclusion
In the realm of software engineering, complexity often begets simplicity. When confronted with the decision of whether to adopt ORM in the context of Node.js, TypeScript, and Express, it’s wise to consider a more streamlined approach. Instead of embracing ORM, consider these insights:
-
Prioritize Performance: For projects with demanding performance requirements, especially those involving intricate queries or high transaction volumes, opting for raw SQL or a database-specific library can lead to more efficient outcomes.
-
Embrace Database Expertise: If your team is well-versed in SQL and the intricacies of the chosen database, utilizing that expertise directly can lead to more optimized database interactions.
-
Maintain Control: When granular control over SQL queries is imperative, using ORM may limit your flexibility. Crafting custom SQL queries allows you to fine-tune operations for optimal performance.
In essence, the decision not to use ORM can be a strategic one, particularly when project goals align with performance, database expertise, and query control. So, when navigating the ORM dilemma, consider a more direct route to achieve your project’s objectives.