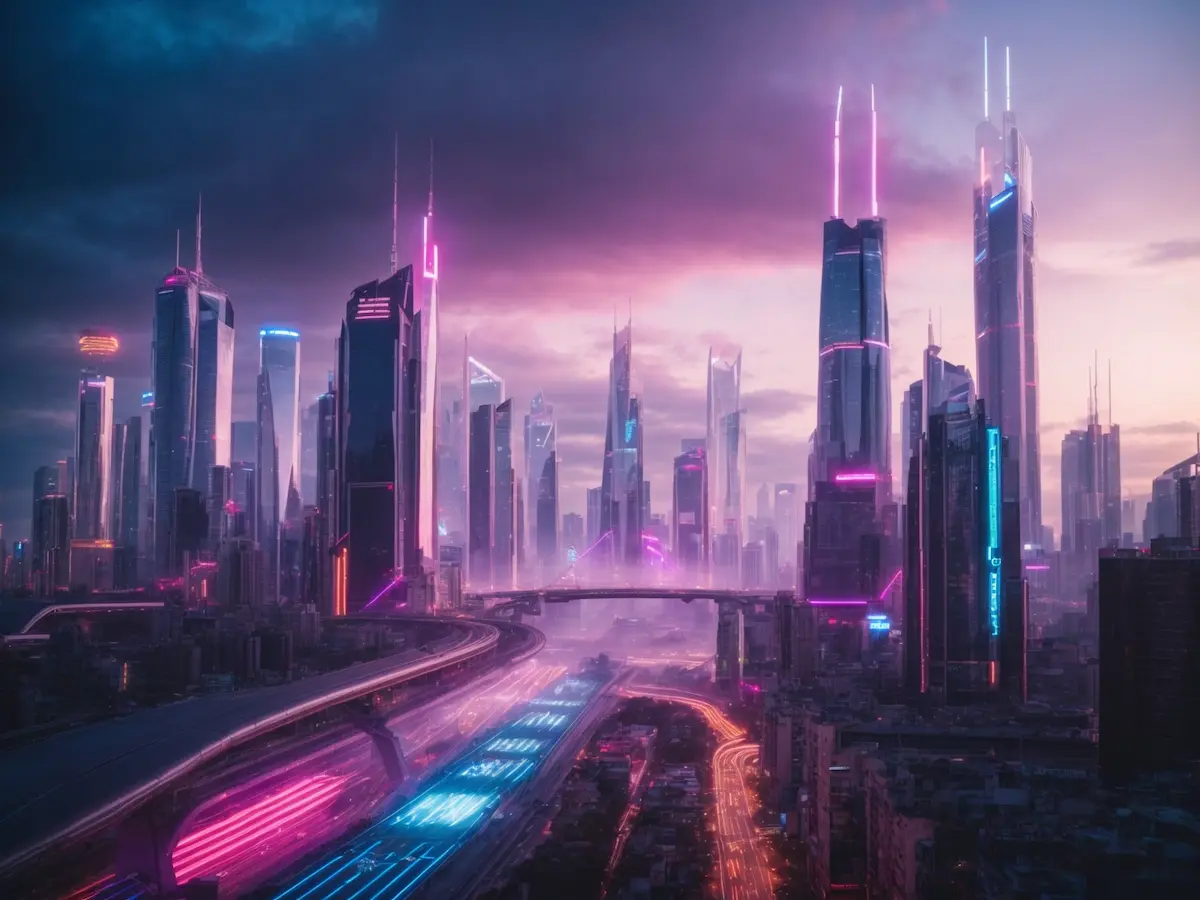
Building a Code Generative AI Model: Empowering Code Writing with AI
- Mohammad Abu Mattar
- Programming , AI
- 29 Aug, 2023
- 05 Mins read
Introduction
In the ever-evolving landscape of software engineering, automation stands as a cornerstone. As a software engineer, have you ever envisioned having an AI companion capable of crafting code snippets, lightening your workload? The good news is that this vision is no longer confined to dreams. Thanks to the emergence of Code Generative AI, you can now tap into the potential of artificial intelligence to write code on your behalf. In this article, we will embark on a journey to construct your very own Code Generative AI model while addressing some pertinent questions along the way.
Can Generative AI Write Code?
Before we delve into the intricacies, let’s confront a fundamental question: can Generative AI genuinely write code? In short, yes, it can. Generative AI models, particularly those founded on neural networks, have demonstrated astonishing capabilities in generating human-like text, including code. These models undergo rigorous training on extensive datasets encompassing various programming languages, enabling them to produce code snippets that are not only syntactically accurate but also semantically meaningful.
What is Generative AI Computer Code?
Generative AI computer code refers to code generated by artificial intelligence models, such as neural networks, using natural language prompts. These models have acquired the nuances and structures of code through extensive training data, enabling them to produce code that closely resembles what a human programmer might write. This generated code can encompass anything from simple functions to intricate algorithms, contingent on the prompt and the model’s training.
How Do I Create a Generative AI for Code?
Now, let’s venture into the practical steps involved in constructing your Code Generative AI model. We will dissect the process step by step, making it accessible for you to create your very own AI code-writing assistant.
Step 1: Environment Setup
To commence your journey, you’ll need a Python environment equipped with the requisite libraries and dependencies. In the following code snippet, we have laid out a fundamental setup for your AI model, complete with imports and configuration settings:
1import logging2import torch3import peft4import transformers5from transformers import AutoTokenizer, AutoModelForCausalLM6from huggingface_hub.hf_api import HfFolder7
8# Configuration class for the Dumpster Copilot Generative model9class Configuration:10 ACCESS_TOKEN = 'ENTER YOUR HUGGINGFACE ACCESS TOKEN HERE'11 LOAD_IN_8BIT = False12 BASE_MODEL = 'meta-llama/Llama-2-7b-chat-hf'13 LORA_WEIGHTS = 'qblocks/llama2-7b-tiny-codes-code-generation'14 PROMPT = 'Write a Python function to divide 2 numbers and check for division by zero.'15
16# Exception classes for errors loading the model and generating text17class ModelLoadingError(Exception):18 pass19
20class DumpsterCopilotGenerativeError(Exception):21 pass22
23# Model loader class24class ModelLoader:25 @staticmethod26 def load_model() -> tuple:27 try:28 tokenizer = AutoTokenizer.from_pretrained(Configuration.LORA_WEIGHTS)29 model = AutoModelForCausalLM.from_pretrained(30 Configuration.BASE_MODEL,31 device_map='auto',32 torch_dtype=torch.float16,33 load_in_8bit=Configuration.LOAD_IN_8BIT34 )35 model = peft.PeftModel.from_pretrained(model, Configuration.LORA_WEIGHTS)36 return tokenizer, model37 except Exception as e:38 raise ModelLoadingError(f'Error loading tokenizer and model: {str(e)}')
In this code snippet, we’ve imported essential libraries like transformers
and torch
, and we’ve introduced a Configuration
class to house critical settings. You’ll also notice the ModelLoader
class, which is responsible for loading the AI model.
Step 2: Loading Your AI Model
Now that your environment is set up, it’s time to load your Code Generative AI model. In the code snippet, we’ve defined a ModelLoader
class with a load_model
method designed to handle model loading. This method returns a tokenizer and a model instance. Remember to replace 'ENTER YOUR HUGGINGFACE ACCESS TOKEN HERE'
with your actual Hugging Face access token.
Step 3: Generating Code with Your AI
With your model loaded, you’re poised to generate code snippets with your AI assistant. We’ve provided a DumpsterCopilotGenerative
class that streamlines the code generation process based on a provided prompt:
1class DumpsterCopilotGenerative:2 def __init__(self, tokenizer, model):3 self.tokenizer = tokenizer4 self.model = model5
6 def dumpster_copilot_generative(self, prompt: str) -> str:7 try:8 logging.info(f'Generating text for prompt: {prompt}')9
10 generator = transformers.pipeline(11 'text-generation',12 model=self.model,13 tokenizer=self.tokenizer14 )15
16 generation_config = transformers.GenerationConfig(17 temperature=0.4,18 top_p=0.99,19 top_k=40,20 num_beams=2,21 max_new_tokens=400,22 repetition_penalty=1.323 )24
25 t = generator(prompt, generation_config=generation_config)26
27 generated_text = t[0]['generated_text']28
29 logging.info(f'Generated text: {generated_text}')30 return generated_text31 except Exception as e:32 raise DumpsterCopilotGenerativeError(f'Error generating text: {str(e)}')
This class incorporates a dumpster_copilot_generative
method, which accepts a prompt as input and furnishes the generated code as output. The code generated hinges on the provided prompt, so ensure that your prompt is explicit and specific.
Step 4: Running Your Code Generative AI
Now that all the elements are in place, you can set your Code Generative AI model in motion to generate code. Here’s an illustration of how you can achieve this:
1if __name__ == '__main__':2 try:3 if Configuration.ACCESS_TOKEN:4 HfFolder.save_token(Configuration.ACCESS_TOKEN)5
6 logging.info('Initiating the text generation process.')7
8 tokenizer, model = ModelLoader.load_model()9 generator = DumpsterCopilotGenerative(tokenizer, model)10 generated_text = generator.dumpster_copilot_generative(Configuration.PROMPT)11
12 logging.info('Generated text:')13 logging.info(generated_text)14
15 logging.info('Successful completion of the text generation process.')16 except (ModelLoadingError, DumpsterCopilotGenerativeError) as e:17 logging.error(f'An error occurred: {str(e)}')
This central block of code initializes your AI model, generates code grounded in the provided prompt (in this instance, “Write a Python function to divide 2 numbers and check for division by zero.”), and logs the resulting code.
Frequently Asked Questions
Now that you have a foundational grasp of constructing a Code Generative AI model, let’s address some frequently posed questions:
Q1: How does Generative AI comprehend programming languages?
Generative AI models acquire an understanding of programming languages through extensive training on code written in various programming languages. They absorb the syntax, semantics, and patterns of code from diverse datasets, allowing them to generate code that conforms to the conventions of specific programming languages.
Q2: Can Generative AI replace human programmers?
Generative AI can automate specific facets
of coding, such as producing boilerplate code or facilitating code completion. However, it does not replace human programmers. Human expertise remains indispensable for conceiving intricate algorithms, debugging, and making pivotal decisions in the realm of software development.
Q3: Are there ethical concerns related to AI-generated code?
Indeed, there exist ethical concerns associated with AI-generated code. These concerns encompass the potential for bias in the training data and the misuse of AI-generated code for nefarious purposes. It is imperative to employ AI-generated code judiciously and ensure that it aligns with ethical standards.
Q4: What are some practical applications of Code Generative AI?
Code Generative AI finds utility in a myriad of practical applications, including code autocompletion, code refactoring, the generation of documentation, and assistance in code reviews. It can significantly enhance developer productivity and contribute to the enhancement of code quality.
Q5: How can I fine-tune my Code Generative AI model?
Fine-tuning a Code Generative AI model involves training it on specific datasets or within particular domains to enhance its specialization. Existing models can be fine-tuned through the utilization of transfer learning techniques and domain-specific data.
Conclusion
In the domain of software engineering, the integration of AI, particularly Code Generative AI, holds the potential to revolutionize code writing. By following the steps elucidated in this article, you can fashion your very own Code Generative AI model to assist you in your coding pursuits. However, it is crucial to bear in mind that while AI constitutes a potent tool, human expertise and ethical considerations should perpetually guide software development.
The journey of crafting your Code Generative AI is a thrilling one, opening up novel possibilities in the sphere of software engineering. So, are you prepared to embark on this coding voyage with AI as your trusty companion?