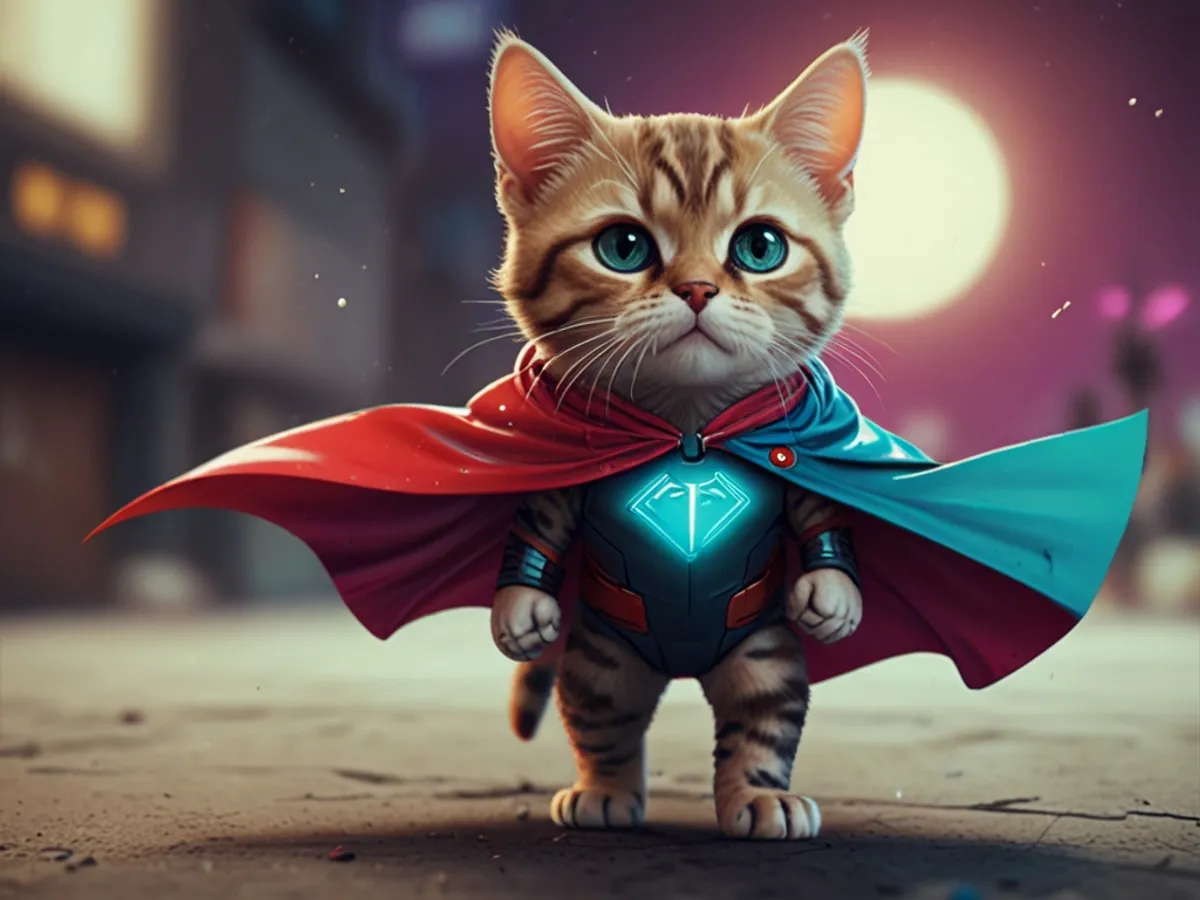
Software Engineering Principles Every Developer Should Know
- Mohammad Abu Mattar
- Software engineering
- Published: 20 May, 2024
- 02 Mins read
In the dynamic world of software development, certain principles stand the test of time, guiding developers towards creating robust, maintainable, and efficient code. Let’s delve into these principles and understand why they are essential for every developer to know.
What Is the DRY Principle and Why Is It Important?
DRY (Don’t Repeat Yourself) is a fundamental principle that emphasizes the importance of code reusability.
- Avoid Code Duplication: Repeating the same code in multiple places increases the risk of errors and makes maintenance challenging.
- Modularize Code: Break down functionality into reusable modules or functions, reducing duplication and promoting consistency.
Here’s an example in Python that doesn’t adhere to the DRY principle, illustrating a common real-world scenario:
def create_user_profile(user_id, name, email): profile = { "id": user_id, "name": name, "email": email, "welcome_message": f"Welcome {name}! Your email is {email}." } print(f"Creating profile for {name} with email {email}") return profile
def send_welcome_email(name, email): message = f"Hello {name}, welcome to our platform! Please verify your email: {email}." print(f"Sending email to {email}: {message}")
The above code repeats the process of constructing welcome messages. Let’s refactor it to adhere to the DRY principle:
def format_welcome_message(name, email): return f"Hello {name}, welcome to our platform! Please verify your email: {email}."
def create_user_profile(user_id, name, email): profile = { "id": user_id, "name": name, "email": email, "welcome_message": format_welcome_message(name, email) } print(f"Creating profile for {name} with email {email}") return profile
def send_welcome_email(name, email): message = format_welcome_message(name, email) print(f"Sending email to {email}: {message}")
By creating a single function to format welcome messages, we eliminate redundancy and improve maintainability.
How Does the KISS Principle Improve Software Development?
KISS (Keep It Simple, Stupid) advocates for simplicity in design and implementation.
- Clarity and Readability: Simple code is easier to understand, debug, and maintain.
- Reduce Complexity: Avoid over-engineering by choosing straightforward solutions over unnecessarily complex ones.
Consider the following Python code snippet for logging user activities:
import logging
def log_user_activity(user_id, activity): logging.basicConfig(level=logging.DEBUG, format='%(asctime)s %(message)s') logger = logging.getLogger() log_message = f"User {user_id} performed {activity}." if activity == 'login': logger.debug(log_message) elif activity == 'logout': logger.debug(log_message) elif activity == 'error': logger.error(log_message) else: logger.info(log_message)
The above code is more complex than necessary. Let’s simplify it:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s %(message)s')logger = logging.getLogger()
def log_user_activity(user_id, activity): log_message = f"User {user_id} performed {activity}." logger.log(logging.DEBUG if activity in ['login', 'logout'] else logging.INFO, log_message)
By using a more straightforward approach, we maintain functionality while enhancing readability and reducing complexity.
What Does YAGNI Mean in Software Development?
YAGNI (You Aren’t Gonna Need It) encourages developers to avoid adding functionality prematurely.
- Focus on Requirements: Implement only the features that are currently needed, avoiding speculative or unnecessary additions.
- Avoid Over-Engineering: By prioritizing essential functionality, developers can minimize complexity and reduce the risk of introducing bugs.
Consider the following Python code snippet for handling user permissions:
def get_user_permissions(user_role, has_admin_rights, is_super_user, is_active): if not is_active: return "No permissions" if is_super_user: return "All permissions" if has_admin_rights: return "Admin permissions" if user_role == "editor": return "Edit permissions" if user_role == "viewer": return "View permissions" return "No permissions"
This code over-engineers the permissions logic. Let’s simplify it by focusing on essential functionality:
def get_user_permissions(user_role): permissions = { "super_user": "All permissions", "admin": "Admin permissions", "editor": "Edit permissions", "viewer": "View permissions" } return permissions.get(user_role, "No permissions")
By adhering to the YAGNI principle, we eliminate unnecessary complexity and focus on core requirements.
Conclusion
Code quality and maintainability can be greatly improved by comprehending and putting software engineering principles like DRY, KISS, and YAGNI into practice. These guidelines help programmers write effective, reliable, and maintainable software by encouraging code reuse, simplicity, and an emphasis on key features.