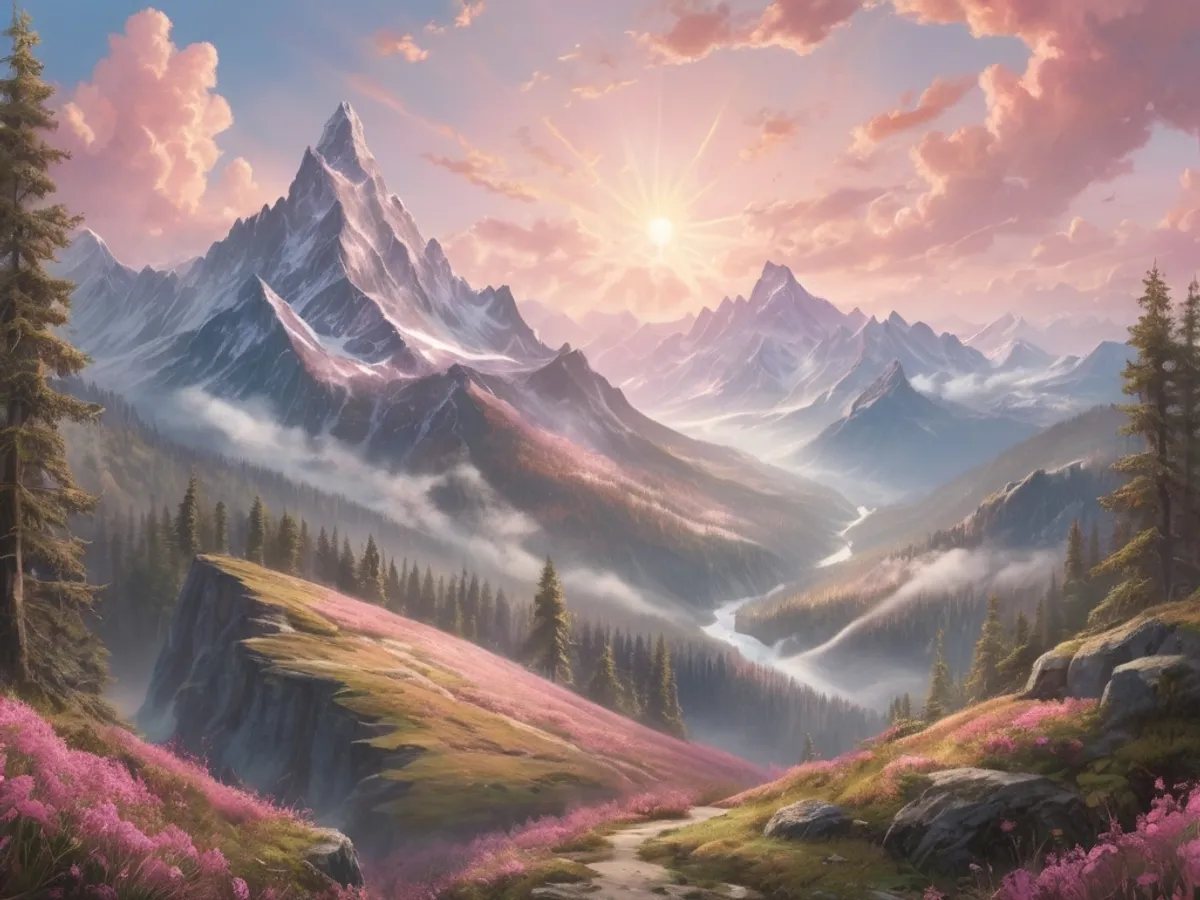
Why You Should Not Use Else Statements in Your Code
- Mohammad Abu Mattar
- Software engineering
- 24 May, 2024
- 03 Mins read
In software engineering, how you structure your code can significantly impact its readability, maintainability, and overall quality. One often-debated topic is the use of else statements. While they seem straightforward, avoiding else statements can lead to cleaner and more understandable code. In this post, we’ll explore why you should reconsider using else statements and introduce some alternative approaches.
What Is a Guard Clause?
Guard clauses are a powerful alternative to else statements. A guard clause is a condition at the beginning of a function that handles special cases or invalid input immediately, allowing the main logic to flow without nested conditions.
Example: Using Guard Clauses
Here’s a simple example in Python to illustrate the concept:
def process_order(order): if order.is_valid(): if order.has_stock(): if order.is_paid(): return "Order processed" else: return "Order not paid" else: return "Out of stock" else: return "Invalid order"
Notice how the nested conditions make the code harder to follow. Now, let’s refactor it using guard clauses:
def process_order(order): if not order.is_valid(): return "Invalid order"
if not order.has_stock(): return "Out of stock"
if not order.is_paid(): return "Order not paid"
return "Order processed"
By handling special cases early, the main logic is clearer and easier to read. Guard clauses reduce indentation and highlight the main flow of the function.
How Does It Establish a Contract?
Establishes a Contract: Using guard clauses can establish a clear contract for your functions. This means defining what conditions must be met for the function to proceed with its main logic.
When you handle edge cases at the beginning, it becomes immediately obvious what preconditions must be satisfied, making the function’s behavior more predictable and understandable.
Example: Establishing a Contract
Consider a function that processes user input:
def process_input(user_input): if user_input is None: raise ValueError("Input cannot be None")
if not isinstance(user_input, str): raise TypeError("Input must be a string")
if user_input == "": raise ValueError("Input cannot be empty")
# Main processing logic return user_input.strip().upper()
By establishing a contract with guard clauses, you ensure that the function’s main logic operates under clearly defined conditions. This approach not only makes the code more readable but also more reliable.
What About Adding New Conditions?
Adding New Conditions: One of the challenges with else statements is that they can lead to deeply nested code, especially when adding new conditions. Guard clauses simplify this by allowing new conditions to be added without increasing indentation levels.
Example: Adding New Conditions with Guard Clauses
Suppose you need to extend the previous order processing function to check for a new condition, such as checking if the order is from a preferred customer:
def process_order(order): if not order.is_valid(): return "Invalid order"
if not order.has_stock(): return "Out of stock"
if not order.is_paid(): return "Order not paid"
if not order.is_preferred_customer(): return "Standard order processing"
return "Priority order processing"
Adding new conditions is straightforward and does not complicate the main logic flow. Each condition is handled explicitly and independently.
When Can You Use Else?
Despite the advantages of avoiding else statements, there are situations where using else can be appropriate, especially when it improves readability or when dealing with mutually exclusive conditions that naturally fall into an if-else pattern.
Example: Appropriate Use of Else
For instance, in a simple function that classifies numbers, using else can make the code more concise and clear:
def classify_number(number): if number > 0: return "Positive" elif number < 0: return "Negative" else: return "Zero"
In this case, the else statement helps to clearly express the mutually exclusive nature of the conditions. The function is simple enough that the else statement doesn’t hinder readability.
Conclusion
Avoiding else statements can lead to cleaner, more maintainable code by leveraging guard clauses and establishing clear contracts for your functions. By handling edge cases upfront and keeping the main logic straightforward, your code becomes easier to read and understand. However, remember that else statements are not inherently bad and can be useful in certain contexts, especially for mutually exclusive conditions.