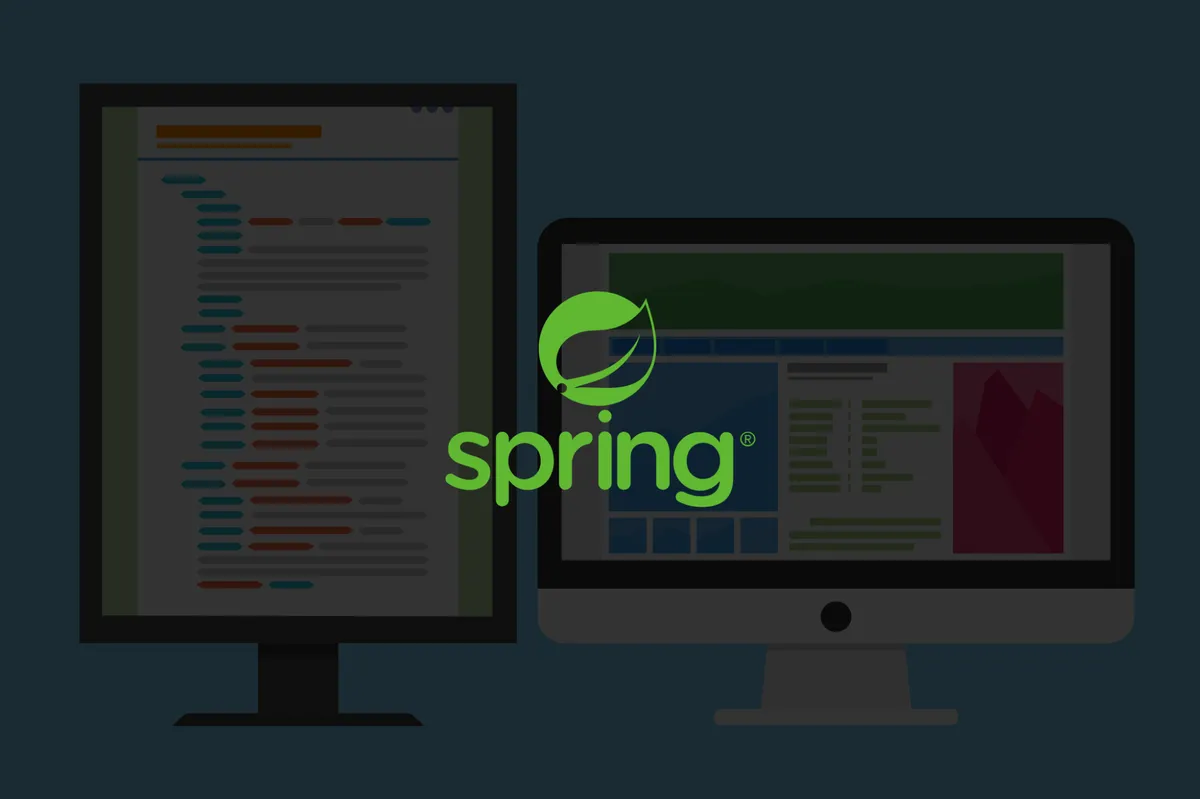
Introduction to Spring Boot Framework
- Mohammad Abu Mattar
- Programming , Backend
- Published: 15 Jan, 2023
- 07 Mins read
Introduction
For creating web apps and microservices, many developers utilize the Spring Boot framework. The fact that it is built on top of the Spring Framework and offers a number of advantages makes it a desirable option for developers. You will learn about Spring Boot in this blog article, as well as why it is such a great tool for creating web apps and how to create a basic Spring Boot application.
What is Spring Boot?
Developers may quickly and simply construct standalone, production-ready apps using the Spring Boot framework. It offers a variety of capabilities, including security, data access, and online services, that may be quickly added to an application. Furthermore, Spring Boot configures itself automatically depending on the dependencies present in the project, doing away with the need for manual configuration.
Why Spring Boot?
Due to its simplicity of use and extensive feature set, Spring Boot is a preferred option for developing web apps and microservices. Developers find it appealing because of its extensive variety of capabilities and capacity to customize itself automatically. Its support for testing and deployment also make it a strong and trustworthy framework for creating web applications.
Spring Boot Features
Easy Setup and Automatic Configuration
One of Spring Boot’s main advantages is its capacity to automatically configure itself in accordance with the dependencies present in the project. As a result, there is no longer a requirement for developers to manually setup the program, which cuts down on the time and effort needed to get an application up and operating.
Stand-Alone Applications
Easy creation and execution of standalone apps is another significant benefit of Spring Boot. This is made possible via the Spring Boot CLI, which enables programmers to rapidly construct a new application by executing a single command.
Web Development
Support for RESTful web services, web sockets, and data validation are just a few of the capabilities that Spring Boot offers for creating online applications. Additionally, it interfaces with a variety of well-known web development tools, like Mustache, FreeMarker, and Thymeleaf, making it simple to build dynamic, interactive web pages.
Testing and Deployment
A variety of features for testing and deploying apps are also offered by Spring Boot. Unit testing tools like JUnit and Mockito are supported by the framework. Additionally, Spring Boot offers a variety of tools, such as metrics and health checks, for administering and monitoring an application once it has been launched.
Build a simple Spring Boot Application
Create a Spring Boot Project
The first step to building a Spring Boot application is to create a new project. This can be done using the Spring Initializer website (https://start.spring.io) or the Spring Boot CLI.
curl https://start.spring.io/starter.tgz \ -d baseDir=spring-boot-web \ -d version=0.0.1-SNAPSHOT \ -d type=maven-project \ -d language=java \ -d bootVersion=2.4.2 \ -d groupId=io.github.mkabumattar \ -d artifactId=spring-boot-web \ -d name=spring-boot-web \ -d packageName=io.github.mkabumattar.springbootweb \ -d dependencies=web \ -d packaging=jar \ -d javaVersion=11 \ -d dependencies=web \ | tar -xzvf -
This command uses curl
to download a starter.tgz
file from the Spring Initializer website, with the specified options passed in as query parameters. The options include:
baseDir
, which sets the base directory for the projectversion
, which sets the version of the projecttype
, which specifies that the project is a Maven projectlanguage
, which sets the programming language of the project as JavabootVersion
, which sets the version of Spring Boot to use in the projectgroupId
, which sets the Maven groupId for the projectartifactId
, which sets the Maven artifactId for the projectname
, which sets the name of the projectpackageName
, which sets the package name for the projectdependencies
, which sets the dependencies needed for the project. In this case, it is webpackaging
, which sets the packaging format as a JAR filejavaVersion
, which sets the Java version to be used in the projectdependencies
, which sets the dependencies needed for the project. In this case, it is web
The output of this command is then piped to tar
command which is used to extract the downloaded file. The options passed to tar
command are xzvf -
which means extract the archive, gzip compressed, verbosely, to stdout.
This command will download and extract a new Spring Boot project with the specified options. The project will have a directory structure that is typical of a Maven project, and it will have the Spring Web dependency already set up and configured.
Create a Controller
The creation of a controller is the next step in handling requests for the application. A Java class called a “controller” is in charge of managing incoming HTTP requests and providing the proper answer. Here is an illustration of a straightforward controller that sends back the JSON object “Hello World”:
package io.github.mkabumattar.springbootweb.controllers;
import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;import java.util.Map;
@RestControllerpublic class HelloWorldController {
@GetMapping("/hello") public Map<String, String> sayHello() { Map<String, String> response = new HashMap<>(); response.put("message", "Hello World!"); return response; }}
The @GetMapping
annotation instructs Spring that this method should handle GET requests to the /hello
endpoint, and the @RestController
annotation instructs Spring that this class should be handled as a REST controller in this example. The function provides a straightforward map with a single key-value pair, with the value “Hello World” and the key “message.”
This is a straightforward example of a Spring Boot controller, but it may be enhanced to handle more complicated routes, accept various requests, and deliver more sophisticated results.
Run the Application
The main method in the produced SpringBootWebApplication.java
file or the spring-boot:run
command (if using the Spring Boot CLI) may be used to launch the application after it has been constructed and a controller has been added.
The program may also be launched by developing it and launching the produced jar file. Use the mvn clean install
command to create a Spring Boot application using Maven, and then use java -jar target/your-jar-file.jar
to launch the created jar file.
When the application is up and running, it will by default be reachable at http://localhost:8080
. By accessing the endpoint http://localhost:8080/hello
in the browser or by sending a GET request to that endpoint using a program like curl or postman, you may test the application. The answer must be a json object with a key-value pair with the value “Hello World” and the key “message.”
By utilizing the server.port
parameter in the application.properties
file or by adding —-server.port=your-port-number>
to the command line arguments when executing the application, you may also select a different port number to use when running the program.
It’s crucial to remember that Spring Boot will automatically establish an embedded Tomcat, Jetty, or Undertow server when you begin the application in order to process web requests and execute your application on it.
Test the Application
After launching the Spring Boot application, you may test it to make sure everything is operating as it should. Making a request to the endpoint(s) specified in the controller(s) using a web browser or a tool like curl
or postman
and observing the outcome is one method of testing the application.
You may test it, for instance, by visiting the URL http://localhost:8080/hello
in a web browser or by sending a GET request to that endpoint using a tool like curl
or postman
. For example, in the preceding example, we created a HelloWorldController
that handles the /hello
endpoint. A json object having a key-value pair, with the value “Hello World” and the key “message,” should be the response.
Making use of a testing framework like JUnit or TestNG, unit tests might be another approach to test the application. The controllers, services, and repository classes are just a few examples of the components of the application that may be tested using these tests. To make it simple to develop tests for a Spring Boot application, Spring Boot offers a variety of annotations and services.
JUnit, a well-liked testing framework for Java applications, can be used as one method of testing a Spring Boot application. Unit tests for specific application parts, such the controllers, services, and repository classes, may be developed using JUnit.
You must include the JUnit dependency in the pom.xml
file of a Spring Boot application that uses Maven as a build tool in order to use JUnit.
Here is an example of how you can add JUnit to your pom.xml
file:
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope></dependency>
JUnit, a well-liked testing framework for Java applications, can be used as one method of testing a Spring Boot application. Unit tests for specific application parts, such the controllers, services, and repository classes, may be developed using JUnit.
Here is an example of how you can use JUnit to test a simple REST controller:
package io.github.mkabumattar.springbootweb.controllers;
import static org.hamcrest.Matchers.is;import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.jsonPath;import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import org.junit.Before;import org.junit.Test;import org.junit.runner.RunWith;import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;import org.springframework.boot.test.context.SpringBootTest;import org.springframework.test.context.junit4.SpringRunner;import org.springframework.test.web.servlet.MockMvc;import org.springframework.test.web.servlet.setup.MockMvcBuilders;
@RunWith(SpringRunner.class)@SpringBootTest@AutoConfigureMockMvcpublic class HelloWorldControllerTest { private MockMvc mockMvc;
@Before public void setUp() { mockMvc = MockMvcBuilders.standaloneSetup(new HelloWorldController()).build(); }
@Test public void testSayHello() throws Exception { mockMvc.perform(get("/hello")) .andExpect(status().isOk()) .andExpect(jsonPath("$.message", is("Hello World!"))); }}
Conclusion
In conclusion, Spring Boot is an effective framework for creating Java web apps. Automatic setup, stand-alone applications, web development, testing, and deployment are just a few of the capabilities it offers to make it simple to set up, configure, and execute a Spring-based application.
We have covered the fundamentals of Spring Boot in this post, along with how to construct a straightforward Spring Boot application. The steps for creating a project, including how to add a Spring Web dependency, a controller, run the application, and test it, have all been demonstrated. Additionally, we spoke about how to test the application using MockMvc and JUnit.
For creating web apps, Spring Boot is a fantastic option since it is simple to get started with and offers a ton of functionality right out of the box. You can discover a lot of tools to help you create and launch your application thanks to its extensive documentation and vast community.
It’s crucial to remember that when it comes to Spring Boot’s possibilities, this is simply the top of the iceberg. You will get more familiar with the framework’s capabilities as you use it more, and you’ll learn how to leverage them to create applications that are more intricate and potent.
References
Here are some references that you can use to learn more about Spring Boot:
- Spring Boot Reference Guide - The official documentation for Spring Boot. This is a comprehensive resource that covers all aspects of the framework.
- Spring Boot in Action - A book by Craig Walls that provides a hands-on introduction to building production-ready Spring Boot applications.
- Spring Boot Starter Projects - A web application that allows you to generate a skeleton project for a Spring Boot application with the dependencies you need.
- Spring Boot on GitHub - The source code and issue tracker for Spring Boot. This is a great place to go to see how the framework is implemented and to find solutions to problems you may encounter.