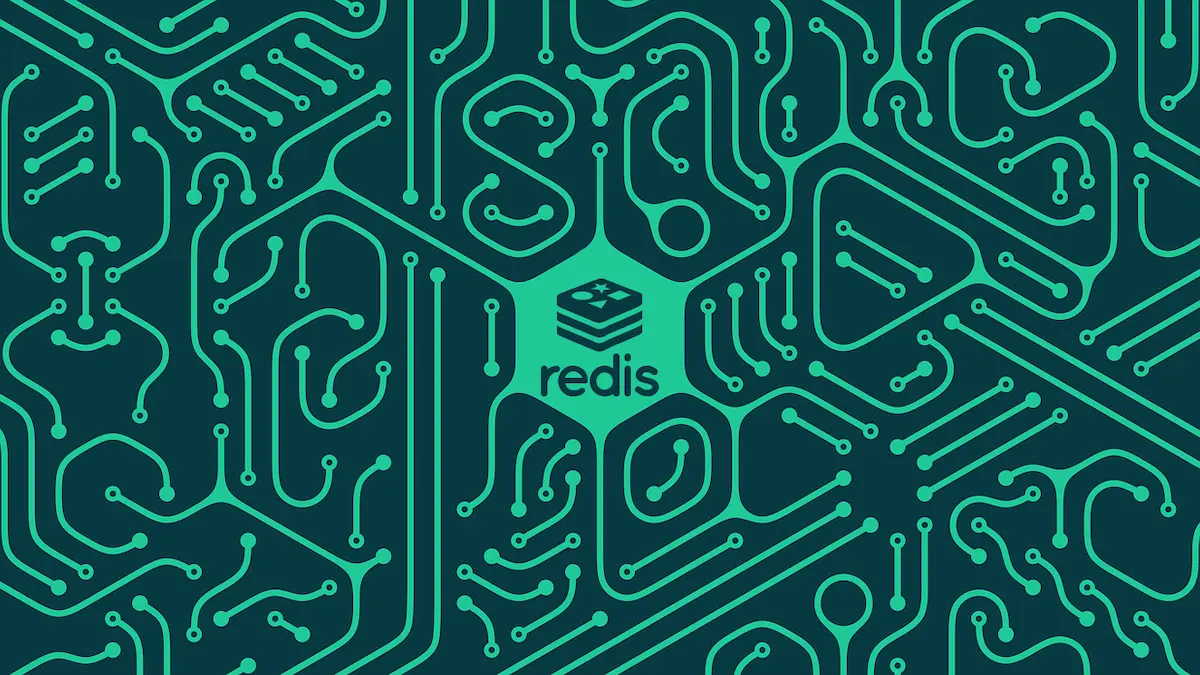
Mastering Caching Strategies with Redis Cache: Boosting Performance in Node.js and TypeScript
- Mohammad Abu Mattar
- Programming , Backend
- 23 Aug, 2023
- 07 Mins read
Introduction
In the ever-evolving realm of software development, the pursuit of optimizing application performance is a perpetual endeavor. Among the arsenal of strategies to attain this goal, the implementation of caching mechanisms stands as a powerful approach. Redis, a high-performing in-memory data store, reigns as a favored choice for caching within Node.js and TypeScript applications. In this comprehensive blog post, we embark on a journey into the intricate realm of caching with Redis. We will explore an array of caching strategies that have the potential to propel your application’s performance to new heights.
Section 1: Grasping Redis as a Caching Solution
Before we plunge into the intricacies of caching strategies, let’s take a moment to fathom why Redis emerges as a stellar choice for caching in Node.js and TypeScript applications. Redis, an open-source in-memory data store, stands renowned for its blistering fast read and write operations. Crafted to handle colossal datasets with minimal latency, Redis presents itself as the ideal candidate for caching frequently accessed data.
Section 2: Unveiling the Redis Cache Key Strategy
An indispensable facet of harnessing Redis’s full potential for caching lies in crafting a robust cache key strategy. The cache key serves as the beacon guiding the retrieval of cached data. It is imperative to forge a key strategy that marries uniqueness with meaningfulness.
Within Redis, keys serve as the gateways to rapid data storage and retrieval. A meticulously constructed cache key strategy wields the power to significantly impact the performance and efficiency of your caching system. Typically, cache keys amalgamate multiple elements, including a namespace, the cached object or data, and any pertinent identifiers. This meticulous approach ensures the keys’ uniqueness, mitigates collisions, and paves the way for effortless data retrieval.
Section 3: A Survey of the Various Caching Types in Redis
Redis extends its support to a spectrum of caching patterns, each tailored to cater to specific use cases. Let’s embark on a journey through some of the most commonly embraced types:
Cache-Aside Pattern
The Cache-Aside pattern, sometimes referred to as Lazy-Loading or Read-Through caching, stands as the simplest caching strategy. Under this pattern, your application code shoulders the responsibility of verifying the cache prior to accessing data from the primary data store, such as a database. If the cache lacks the requisite data, it is procured from the data store and subsequently stashed within the cache for future utilization. This approach, though straightforward, necessitates judicious handling of cache invalidation.
Read-Through Pattern
An evolutionary extension of the Cache-Aside pattern, the Read-Through pattern introduces an abstraction layer between your application and the cache. When your application solicits data, this intermediary layer conducts a preliminary cache check. In the absence of the data within the cache, it retrieves the data from the data store, populates the cache, and subsequently furnishes the data to your application. This methodology streamlines your application code by abstracting cache access.
Write-Through Pattern
In the Write-Through pattern, data undergoes simultaneous storage in both the cache and the primary data store. When your application initiates write or data update operations, the cache is the initial recipient of the data. Subsequently, the data is synchronized with the data store. While this approach introduces a modicum of overhead to write operations, it ensconces the cache with the most up-to-date data at all times.
Write-Behind Pattern
The Write-Behind pattern, also recognized as Write-Behind Caching or Write-Behind Processing, ventures down an alternative route. Within this framework, write operations first transpire within the cache, followed by an asynchronous relay to the primary data store. This approach serves to heighten write performance by mitigating immediate write latency. However, it necessitates vigilant management to preserve data consistency.
Section 4: Navigating the Realm of Popular Caching Strategies
Having embarked on an expedition through the diverse caching patterns, it is imperative to comprehend the contexts in which each strategy radiates.
Cache-Aside Pattern: Simplicity Meets Control
The Cache-Aside pattern proves its mettle when simplicity and meticulous control over cached data are paramount. This approach empowers you to make judicious decisions regarding when to populate the cache, offering direct control over cache invalidation. Nevertheless, it demands judicious programming to ensure data consistency between the cache and the primary data store.
Read-Through Pattern: Abstracting Cache Interaction
The Read-Through pattern steps into the limelight when the goal is to abstract cache interaction from your application’s codebase. By offloading cache management to an abstraction layer, this pattern simplifies your codebase. It finds its niche in applications laden with intricate data access logic, where centralizing caching decisions proves advantageous.
Write-Through Pattern: Upholding Data Consistency
In scenarios where data consistency takes precedence and you are amenable to bearing a marginal overhead in write operations, the Write-Through pattern emerges as the stalwart choice. It guarantees that the cache perpetually houses up-to-the-minute data, rendering it a fitting choice for applications where stale data could prove detrimental.
Write-Behind Pattern: Pinnacle of Write Performance
The Write-Behind pattern shines when the objective is to optimize write performance while permitting a degree of eventual consistency. Through asynchronous data relay to the primary data store, it circumvents immediate write latency, a boon in applications replete with high write loads.
Section 5: Breathing Life into Caching in Node.js and TypeScript with Redis
With a firm grasp of caching strategies, it’s time to venture into the realm of implementation within Node.js and TypeScript using Redis.
Initiating Redis in Node.js
To commence this journey, you must equip yourself with the Redis client library tailored for Node.js. The ioredis
library, available in both Promise-based and callback-based variants, stands as a favored choice. Installation is effortlessly accomplished through npm or yarn:
npm install ioredis# oryarn add ioredis
With the library in place, you gain the capability to establish a connection with your Redis instance, setting the stage for caching.
Embarking on Cache-Aside in Node.js
To breathe life into Cache-Aside within Node.js, your code must be poised to conduct cache checks prior to data store access. The following snippet, employing the ioredis
library, provides a streamlined example:
import Redis from 'ioredis';
// Instantiate a Redis clientconst redis = new Redis();
// Define a function to retrieve data from either the cache or the data storeasync function getDataFromCacheOrStore(key: string) { // Check the cache for the desired data const cachedData = await redis.get(key);
// If the cache yields the data, return it if (cachedData) { return cachedData; }
// Otherwise, retrieve the data from the data store const dataFromStore = await fetchDataFromStore(key);
// Populate the cache with the data for future use await redis.set(key, JSON.stringify(dataFromStore));
// Return the data return dataFromStore;}
This code employs an initial cache check for the requested data. In the absence of the data within the cache, it forages from the data store,
deposits it within the cache, and eventually delivers it.
Embarking on Read-Through in Node.js
To embrace the Read-Through pattern within Node.js, you must erect an abstraction layer responsible for managing both cache and data store interactions. The ensuing code illustrates this concept:
import Redis from 'ioredis';
// Instantiate a Redis clientconst redis = new Redis();
// Define a function to retrieve data, abstracting cache and data store interactionsasync function getData(key: string) { // Fetch data from the cache const cachedData = await redis.get(key);
// Furnish the data if found within the cache if (cachedData) { return JSON.parse(cachedData); }
// Retrieve the data from the data store const dataFromStore = await fetchDataFromStore(key);
// Populate the cache with the fetched data await redis.set(key, JSON.stringify(dataFromStore));
// Return the data return dataFromStore;}
In this code, the getData
function assumes the role of an intermediary, shielding your application code from the intricacies of cache and data store access.
Embarking on Write-Through in Node.js
To set the wheels in motion for the Write-Through pattern within Node.js, it becomes imperative to adapt your write operations to encompass both cache and data store updates. The following code snippet elucidates this process:
import Redis from 'ioredis';
// Instantiate a Redis clientconst redis = new Redis();
// Define a function to update data, ensuring synchronization between cache and data storeasync function updateData(key: string, newData: Record<string, unknown>) { // Prioritize cache update await redis.set(key, JSON.stringify(newData));
// Subsequently, update the data store await updateDataStore(key, newData);}
In this code, the updateData
function orchestrates atomic updates within the cache and data store, preserving data consistency.
Embarking on Write-Behind in Node.js
The Write-Behind pattern within Node.js introduces intricacies due to its asynchronous data store writes. The ensuing code fragment offers an insight into its implementation:
import Redis from 'ioredis';
// Instantiate a Redis clientconst redis = new Redis();
// Define a function to update data, prioritizing cache updates and deferring data store updates asynchronouslyasync function updateData(key: string, newData: Record<string, unknown>) { // Initiate cache update await redis.set(key, JSON.stringify(newData));
// Confer data store update asynchronously without awaiting its completion updateDataStore(key, newData);}
Within this code, the updateData
function instantiates an immediate cache update, followed by the asynchronous relay of data to the primary data store. This asynchronous facet endeavors to augment write performance, particularly in scenarios where immediate data store writes assume a less critical role.
Section 6: Harnessing Advanced Redis Features for Caching
Redis encompasses advanced features that hold the potential to elevate your caching strategies to greater heights. Among these features are:
Expiration Policies
Redis extends the capability to set expiration times for keys. This feature proves invaluable when the goal is to safeguard cached data against staleness. By configuring an appropriate expiration time, you can automate the removal of outdated data from the cache.
Pub/Sub Messaging
Redis boasts support for Publish/Subscribe (Pub/Sub) messaging, a feature that can be harnessed to broadcast notifications to multiple components within your application when data undergoes alterations. This capability becomes a potent asset in scenarios necessitating real-time updates.
Lua Scripting
Redis grants the privilege of executing Lua scripts directly on the server. This feature serves as a formidable tool for the implementation of intricate caching logic, atomic updates across multiple keys, and other advanced functionalities.
Section 7: Scaling Redis for Caching in Expansive Applications
As your application undergoes expansion, the need to scale your Redis caching infrastructure becomes evident. Redis provides support for clustering and sharding, mechanisms that facilitate the dispersion of data across multiple Redis instances. This approach ensures not only high availability but also heightened performance, serving as a robust solution for your caching needs.
Section 8: Is Redis an Apt Choice for Caching?
A frequently posed question centers on the suitability of Redis as a caching solution. In the majority of cases, Redis emerges as an exemplary choice, thanks to its exceptional performance, adaptability, and the gamut of caching patterns it accommodates. Nevertheless, it is imperative to meticulously weigh your specific use case and prerequisites. In scenarios characterized by exorbitant read and write loads, fine-tuning your Redis configuration may become a necessity, and exploration of alternative caching solutions may merit consideration.
Section 9: Conclusion
In conclusion, the mastery of caching strategies within the realm of Redis can wield a profound impact on the performance of your Node.js and TypeScript applications. By acquainting yourself with the Cache-Aside, Read-Through, Write-Through, and Write-Behind patterns, and by instating a robust Redis cache key strategy, you stand poised to unleash the full potential of Redis as a caching powerhouse. Whether you are crafting a diminutive web application or steering the helm of a sprawling, enterprise-scale system, Redis assumes the mantle of an invaluable tool for optimizing data access and elevating user experiences.
Redis, with its innate simplicity, blazing speed, and a treasure trove of advanced features, stakes its claim as a premier choice for caching within the modern landscape of application development. So, without further ado, embark on this journey, experiment with caching patterns within your Node.js and TypeScript projects, and unleash the prowess of Redis to supercharge your applications.