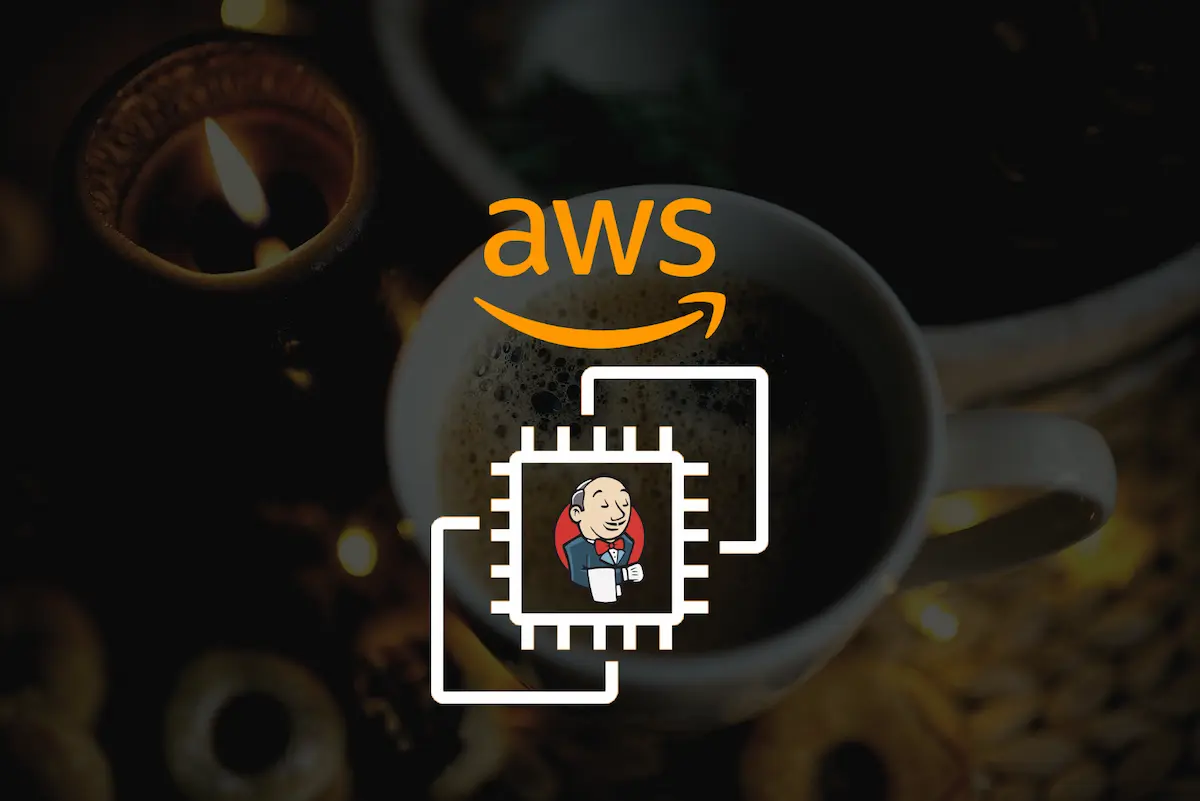
How to CI/CD AWS With Github using Jenkins
- Mohammad Abu Mattar
- Cloud Computing , DevOps
- 07 Dec, 2022
- 13 Mins read
Introduction
In previous posts, I have shown you how to setup Jenkins on AWS EC2 instance. You can check the post here.
In this post, I will show you how to setup a CI/CD pipeline using Jenkins and Github to deploy a simple PHP application to Devlopment and Production environments on AWS. With this setup, you can deploy your application to AWS with a single click.
Prerequisites
- AWS CLI installed and configured
- IAM user with the following permissions:
- AmazonVPCFullAccess
- AmazonEC2FullAccess
Setup AWS Infrastructure for Devlopment Environment
Create VPC
Step 1: Create VPC
To create a VPC, run the following command:
# Create a VPCAWS_VPC=$(aws ec2 create-vpc \ --cidr-block 10.0.0.0/16 \ --query 'Vpc.VpcId' \ --output text)
# Add a name tag to the VPCaws ec2 create-tags \ --resources $AWS_VPC \ --tags Key=Name,Value=environments-vpc
Step 2: Modify your custom VPC and enable DNS hostname support, and DNS support
To modify your custom VPC and enable DNS hostname support, and DNS support, run the following command:
# Modify your custom VPC and enable DNS hostname support, and DNS support# Enable DNS hostnamesaws ec2 modify-vpc-attribute \ --vpc-id $AWS_VPC \ --enable-dns-hostnames "{\"Value\":true}"
# Enable DNS supportaws ec2 modify-vpc-attribute \ --vpc-id $AWS_VPC \ --enable-dns-support "{\"Value\":true}"
Step 3: Create a Public Subnet
To create a public subnet, run the following command:
# Create a public subnetAWS_PUBLIC_SUBNET=$(aws ec2 create-subnet \ --vpc-id $AWS_VPC \ --cidr-block 10.0.1.0/24 \ --query 'Subnet.SubnetId' \ --output text)
# Add a name tag to the public subnetaws ec2 create-tags \ --resources $AWS_PUBLIC_SUBNET \ --tags Key=Name,Value=environments-public-subnet
Step 4: Enable Auto-assign Public IP on the subnet
To enable auto-assign public IP on the subnet, run the following command:
# Enable auto-assign public IP on the subnetaws ec2 modify-subnet-attribute \ --subnet-id $AWS_PUBLIC_SUBNET \ --map-public-ip-on-launch
Step 5: Create an Internet Gateway
To create an internet gateway, run the following command:
# Create an Internet GatewayAWS_INTERNET_GATEWAY=$(aws ec2 create-internet-gateway \ --query 'InternetGateway.InternetGatewayId' \ --output text)
# Add a name tag to the Internet Gatewayaws ec2 create-tags \ --resources $AWS_INTERNET_GATEWAY \ --tags Key=Name,Value=environments-internet-gateway
Step 6: Attach the Internet Gateway to your VPC
To attach the internet gateway to your VPC, run the following command:
# Attach the Internet Gateway to the VPCaws ec2 attach-internet-gateway \ --internet-gateway-id $AWS_INTERNET_GATEWAY \ --vpc-id $AWS_VPC
Step 7: Create a Route Table
To create a route table, run the following command:
# Create a route tableAWS_ROUTE_TABLE=$(aws ec2 create-route-table \ --vpc-id $AWS_VPC \ --query 'RouteTable.RouteTableId' \ --output text)
# Add a name tag to the route tableaws ec2 create-tags \ --resources $AWS_ROUTE_TABLE \ --tags Key=Name,Value=environments-route-table
Step 8: Create a custom route table association
To create a route in the route table, run the following command:
# Create a custom route table associationaws ec2 associate-route-table \ --subnet-id $AWS_PUBLIC_SUBNET \ --route-table-id $AWS_ROUTE_TABLE
Step 9: Associate the subnet with route table, making it a public subnet
To associate the subnet with route table, making it a public subnet, run the following command:
# Associate the subnet with route table, making it a public subnetaws ec2 create-route \ --route-table-id $AWS_ROUTE_TABLE \ --destination-cidr-block 0.0.0.0/0 \ --gateway-id $AWS_INTERNET_GATEWAY
Step 10: Create a Security Group
To create a security group, run the following command:
# Create a security groupAWS_SECURITY_GROUP=$(aws ec2 create-security-group \ --group-name aws-security-group \ --description "AWS Security Group" \ --vpc-id $AWS_VPC \ --query 'GroupId' \ --output text)
# Add a name tag to the security groupaws ec2 create-tags \ --resources $AWS_SECURITY_GROUP \ --tags Key=Name,Value=environments-security-group
Step 11: Add inbound rules to the security group
To add inbound rules to the security group, run the following command:
# Add inbound rules to the security group
# Add SSH ruleaws ec2 authorize-security-group-ingress \ --group-id $AWS_SECURITY_GROUP \ --protocol tcp \ --port 22 \ --cidr 0.0.0.0/0 \ --output text
# Add HTTP ruleaws ec2 authorize-security-group-ingress \ --group-id $AWS_SECURITY_GROUP \ --protocol tcp \ --port 80 \ --cidr 0.0.0.0/0 \ --output text
# Add HTTPS ruleaws ec2 authorize-security-group-ingress \ --group-id $AWS_SECURITY_GROUP \ --protocol tcp \ --port 443 \ --cidr 0.0.0.0/0 \ --output text
Create a Two EC2 Instances
Step 1: Get the latest AMI ID for Amazon Linux 2
To get the latest AMI ID for Amazon Linux 2, run the following command:
# Get the latest AMI ID for Amazon Linux 2AWS_AMI=$(aws ec2 describe-images \ --owners 'amazon' \ --filters 'Name=name,Values=amzn2-ami-hvm-2.0.*' \ 'Name=state,Values=available' \ --query 'sort_by(Images, &CreationDate)[-1].[ImageId]' \ --output 'text')
Step 2: Create a Key Pair
To create a key pair, run the following command:
# Create a key pairaws ec2 create-key-pair \ --key-name aws-key-pair \ --query 'KeyMaterial' \ --output text > aws-key-pair.pem
# Change the permission of the key pairchmod 400 aws-key-pair.pem
Step 3: Create a user data script
To create a user data script, run the following command:
# Create a userdata scriptcat <<EOF > userdata.sh#!/bin/bash
# update the systemsudo yum update -y
# install httpdsudo yum install -y httpd
# start httpdsudo systemctl start httpd
# enable httpdsudo systemctl enable httpd
# at first, we will enable amazon-linux-extras so that we can specify the PHP version that we want to install.sudo amazon-linux-extras enable php7.4 -y
# install phpsudo yum install -y php php-{pear,cgi,common,curl,mbstring,gd,mysqlnd,gettext,bcmath,json,xml,fpm,intl,zip,imap}
# install MariaDBsudo yum install -y mariadb-server
# start MariaDBsudo systemctl start mariadb
# enable MariaDBsudo systemctl enable mariadb
# we will now secure MariaDB.sudo mysql_secure_installation <<EOF2y121612121612yyyyEOF2
# change the ownership of the /var/www directory to the apache user and group.sudo chown -R apache:apache /var/www
# this will give read, write, and execute permissions to the owner, group, and others.sudo chmod -R 775 /var/www
# this is for the wp-config.php filesudo chmod -R 755 /var/www/html
# restart MariaDBsudo systemctl restart mariadb
# restart httpdsudo systemctl restart httpdEOF
Step 4: Create an EC2 instance
To create an EC2 instance, run the following command:
# Create an EC2 InstanceAWS_EC2_INSTANCE_PROD=$(aws ec2 run-instances \ --image-id $AWS_AMI \ --count 1 \ --instance-type t2.micro \ --key-name aws-key-pair \ --security-group-ids $AWS_SECURITY_GROUP \ --subnet-id $AWS_PUBLIC_SUBNET \ --user-data file://userdata.sh \ --query 'Instances[0].InstanceId' \ --output text)
AWS_EC2_INSTANCE_DEV=$(aws ec2 run-instances \ --image-id $AWS_AMI \ --count 1 \ --instance-type t2.micro \ --key-name aws-key-pair \ --security-group-ids $AWS_SECURITY_GROUP \ --subnet-id $AWS_PUBLIC_SUBNET \ --user-data file://userdata.sh \ --query 'Instances[0].InstanceId' \ --output text)
# Add a name tag to the EC2 Instanceaws ec2 create-tags \ --resources $AWS_EC2_INSTANCE_PROD \ --tags Key=Name,Value=environments-prod
aws ec2 create-tags \ --resources $AWS_EC2_INSTANCE_DEV \ --tags Key=Name,Value=environments-dev
Step 5: Create an Elastic IP
To create an Elastic IP, run the following command:
# Create an Elastic IPAWS_ELASTIC_IP_PROD=$(aws ec2 allocate-address \ --domain vpc \ --query 'AllocationId' \ --output text)
AWS_ELASTIC_IP_DEV=$(aws ec2 allocate-address \ --domain vpc \ --query 'AllocationId' \ --output text)
# Add a name tag to the Elastic IPaws ec2 create-tags \ --resources $AWS_ELASTIC_IP_PROD \ --tags Key=Name,Value=environments-prod
aws ec2 create-tags \ --resources $AWS_ELASTIC_IP_DEV \ --tags Key=Name,Value=environments-dev
Step 6: Associate the Elastic IP with the EC2 Instance
To associate the Elastic IP with the EC2 Instance, run the following command:
# Associate the Elastic IP with the EC2 Instanceaws ec2 associate-address \ --instance-id $AWS_EC2_INSTANCE_PROD \ --allocation-id $AWS_ELASTIC_IP_PROD
aws ec2 associate-address \ --instance-id $AWS_EC2_INSTANCE_DEV \ --allocation-id $AWS_ELASTIC_IP_DEV
Step 7: Get the Public IP Address of the EC2 Instance
To get the public IP address of the EC2 Instance, run the following command:
# Get the public IP address of the EC2 InstanceAWS_PUBLIC_IP_PROD=$(aws ec2 describe-instances \ --instance-ids $AWS_EC2_INSTANCE_PROD \ --query 'Reservations[0].Instances[0].PublicIpAddress' \ --output text)
AWS_PUBLIC_IP_DEV=$(aws ec2 describe-instances \ --instance-ids $AWS_EC2_INSTANCE_DEV \ --query 'Reservations[0].Instances[0].PublicIpAddress' \ --output text)
Setup Simple PHP Application
Create a GitHub Repository
Step 1: Create a GitHub repository
To create a GitHub repository, run the following command:
# Create a GitHub repositorycurl -u $GITHUB_USERNAME https://api.github.com/user/repos -d '{"name":"quick-test-jenkins"}'
or you can create a repository from the GitHub website, go to Create a new repository and create a new repository.
- Repository name: quick-test-jenkins
- Description: Quick Test Jenkins
- Public/Private: Private
- Initialize this repository with a README: No
- Add .gitignore: None
- Add a license: None
Step 2: Clone the GitHub repository
To clone the GitHub repository, run the following command:
# Clone the GitHub repositorygit clone git@github.com:MKAbuMattar/quick-test-jenkins.git
# Change directorycd quick-test-jenkins
Step 3: Create a new branch
To create a new branch, run the following command:
# Create a new branchgit checkout -b devlopment
Create a Simple PHP Application
Step 1: Create the structure of the application
To create the structure of the application, run the following command:
# Create the structure of the applicationmkdir -p app/{config,controllers,views} assets/{css}
Step 2: Create the env.php
file
To create the config.php
file, run the following command:
1<?php2
3// Define the environment4define('URL', $_SERVER['HTTP_HOST']);5define('DEV_DNS', 'DEVLOPMENT_DNS');6define('PROD_DNS', 'PRODUCTION_DNS');7define('DEV', 'DEVLOPMENT_IP');8define('PROD', 'PRODUCTION_IP');9
10// check the environment11if (URL == PROD_DNS || URL == PROD) {12 define('ENV', 'PROD');13} elseif (URL == DEV_DNS || URL == DEV) {14 define('ENV', 'DEV');15} else {16 define('ENV', 'LOCAL');17}18
19
20// Define the database21switch (ENV) {22 case 'PROD':23 define( 'DB_NAME', 'PROD_PHP');24 define( 'DB_USER', 'PROD_USER' );25 define( 'DB_PASSWORD', '161216' );26 define( 'DB_HOST', 'localhost' );27 break;28 case 'DEV':29 define( 'DB_NAME', 'DEV_PHP');30 define( 'DB_USER', 'DEV_USER' );31 define( 'DB_PASSWORD', '121612' );32 define( 'DB_HOST', 'localhost' );33 break;34 default:35 define( 'DB_NAME', '');36 define( 'DB_USER', '' );37 define( 'DB_PASSWORD', '' );38 define( 'DB_HOST', '' );39 break;40}
Step 3: Create the connection.php
file
To create the connection.php
file, run the following command:
1<?php2
3// Include the env.php file4require_once 'env.php';5
6// Create a connection to the database7try {8 $conn = new PDO("mysql:host=".DB_HOST.";dbname=".DB_NAME, DB_USER, DB_PASSWORD);9 $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);10} catch (PDOException $e) {11 echo "Connection failed: " . $e->getMessage();12}
Step 4: Create the controller file
To create the controller file, run the following command:
1<?php2
3// Include the connection.php file4require_once 'app/config/connection.php';5
6// Get the data from the database7$query = "SELECT * FROM `users`";8$stmt = $conn->prepare($query);9$stmt->execute();10$users = $stmt->fetchAll(PDO::FETCH_ASSOC);11
12// close the connection13$conn = null;
Step 5: Create the view file
To create the view file, run the following command:
1<!DOCTYPE html>2<html>3 <head>4 <meta charset="utf-8" />5 <meta name="viewport" content="width=device-width, initial-scale=1" />6 <title><?php echo ENV; ?> | My Site</title>7 <link rel="stylesheet" href="./assets/css/normalize.css" />8 <link rel="stylesheet" href="./assets/css/main.css" />9 </head>10 <body>11 <header>12 <h1>My Site</h1>13
14 <nav>15 <ul>16 <li><a href="./index.php">Home</a></li>17 </ul>18 </nav>19 </header>20
21 <main>22 <h2>Home</h2>23 <p>Welcome to my site!</p>24 <p>25 <?php echo "Environment: " . ENV; ?>26 </p>27 </main>28
29 <footer>30 <p>My Site © 2022</p>31 </footer>32 </body>33</html>
Step 6: Create the index.php
file
To create the index.php
file, run the following command:
1<?php2
3// Include the controller file4require_once 'app/controllers/index.php';5
6// Include the view file7require_once 'app/views/index.php';
Step 7: Create the normalize.css
file
To create the normalize.css
file, run the following command:
Click to see the code of the `normalize.css` file
1/*! normalize.css v8.0.1 | MIT License | github.com/necolas/normalize.css */2
3/* Document4 ========================================================================== */5
6/**7 * 1. Correct the line height in all browsers.8 * 2. Prevent adjustments of font size after orientation changes in iOS.9 */10
11html {12 line-height: 1.15; /* 1 */13 -webkit-text-size-adjust: 100%; /* 2 */14}15
16/* Sections17 ========================================================================== */18
19/**20 * Remove the margin in all browsers.21 */22
23body {24 margin: 0;25}26
27/**28 * Render the `main` element consistently in IE.29 */30
31main {32 display: block;33}34
35/**36 * Correct the font size and margin on `h1` elements within `section` and37 * `article` contexts in Chrome, Firefox, and Safari.38 */39
40h1 {41 font-size: 2em;42 margin: 0.67em 0;43}44
45/* Grouping content46 ========================================================================== */47
48/**49 * 1. Add the correct box sizing in Firefox.50 * 2. Show the overflow in Edge and IE.51 */52
53hr {54 box-sizing: content-box; /* 1 */55 height: 0; /* 1 */56 overflow: visible; /* 2 */57}58
59/**60 * 1. Correct the inheritance and scaling of font size in all browsers.61 * 2. Correct the odd `em` font sizing in all browsers.62 */63
64pre {65 font-family: monospace, monospace; /* 1 */66 font-size: 1em; /* 2 */67}68
69/* Text-level semantics70 ========================================================================== */71
72/**73 * Remove the gray background on active links in IE 10.74 */75
76a {77 background-color: transparent;78}79
80/**81 * 1. Remove the bottom border in Chrome 57-82 * 2. Add the correct text decoration in Chrome, Edge, IE, Opera, and Safari.83 */84
85abbr[title] {86 border-bottom: none; /* 1 */87 text-decoration: underline; /* 2 */88 text-decoration: underline dotted; /* 2 */89}90
91/**92 * Add the correct font weight in Chrome, Edge, and Safari.93 */94
95b,96strong {97 font-weight: bolder;98}99
100/**101 * 1. Correct the inheritance and scaling of font size in all browsers.102 * 2. Correct the odd `em` font sizing in all browsers.103 */104
105code,106kbd,107samp {108 font-family: monospace, monospace; /* 1 */109 font-size: 1em; /* 2 */110}111
112/**113 * Add the correct font size in all browsers.114 */115
116small {117 font-size: 80%;118}119
120/**121 * Prevent `sub` and `sup` elements from affecting the line height in122 * all browsers.123 */124
125sub,126sup {127 font-size: 75%;128 line-height: 0;129 position: relative;130 vertical-align: baseline;131}132
133sub {134 bottom: -0.25em;135}136
137sup {138 top: -0.5em;139}140
141/* Embedded content142 ========================================================================== */143
144/**145 * Remove the border on images inside links in IE 10.146 */147
148img {149 border-style: none;150}151
152/* Forms153 ========================================================================== */154
155/**156 * 1. Change the font styles in all browsers.157 * 2. Remove the margin in Firefox and Safari.158 */159
160button,161input,162optgroup,163select,164textarea {165 font-family: inherit; /* 1 */166 font-size: 100%; /* 1 */167 line-height: 1.15; /* 1 */168 margin: 0; /* 2 */169}170
171/**172 * Show the overflow in IE.173 * 1. Show the overflow in Edge.174 */175
176button,177input {178 /* 1 */179 overflow: visible;180}181
182/**183 * Remove the inheritance of text transform in Edge, Firefox, and IE.184 * 1. Remove the inheritance of text transform in Firefox.185 */186
187button,188select {189 /* 1 */190 text-transform: none;191}192
193/**194 * Correct the inability to style clickable types in iOS and Safari.195 */196
197button,198[type="button"],199[type="reset"],200[type="submit"] {201 -webkit-appearance: button;202}203
204/**205 * Remove the inner border and padding in Firefox.206 */207
208button::-moz-focus-inner,209[type="button"]::-moz-focus-inner,210[type="reset"]::-moz-focus-inner,211[type="submit"]::-moz-focus-inner {212 border-style: none;213 padding: 0;214}215
216/**217 * Restore the focus styles unset by the previous rule.218 */219
220button:-moz-focusring,221[type="button"]:-moz-focusring,222[type="reset"]:-moz-focusring,223[type="submit"]:-moz-focusring {224 outline: 1px dotted ButtonText;225}226
227/**228 * Correct the padding in Firefox.229 */230
231fieldset {232 padding: 0.35em 0.75em 0.625em;233}234
235/**236 * 1. Correct the text wrapping in Edge and IE.237 * 2. Correct the color inheritance from `fieldset` elements in IE.238 * 3. Remove the padding so developers are not caught out when they zero out239 * `fieldset` elements in all browsers.240 */241
242legend {243 box-sizing: border-box; /* 1 */244 color: inherit; /* 2 */245 display: table; /* 1 */246 max-width: 100%; /* 1 */247 padding: 0; /* 3 */248 white-space: normal; /* 1 */249}250
251/**252 * Add the correct vertical alignment in Chrome, Firefox, and Opera.253 */254
255progress {256 vertical-align: baseline;257}258
259/**260 * Remove the default vertical scrollbar in IE 10+.261 */262
263textarea {264 overflow: auto;265}266
267/**268 * 1. Add the correct box sizing in IE 10.269 * 2. Remove the padding in IE 10.270 */271
272[type="checkbox"],273[type="radio"] {274 box-sizing: border-box; /* 1 */275 padding: 0; /* 2 */276}277
278/**279 * Correct the cursor style of increment and decrement buttons in Chrome.280 */281
282[type="number"]::-webkit-inner-spin-button,283[type="number"]::-webkit-outer-spin-button {284 height: auto;285}286
287/**288 * 1. Correct the odd appearance in Chrome and Safari.289 * 2. Correct the outline style in Safari.290 */291
292[type="search"] {293 -webkit-appearance: textfield; /* 1 */294 outline-offset: -2px; /* 2 */295}296
297/**298 * Remove the inner padding in Chrome and Safari on macOS.299 */300
301[type="search"]::-webkit-search-decoration {302 -webkit-appearance: none;303}304
305/**306 * 1. Correct the inability to style clickable types in iOS and Safari.307 * 2. Change font properties to `inherit` in Safari.308 */309
310::-webkit-file-upload-button {311 -webkit-appearance: button; /* 1 */312 font: inherit; /* 2 */313}314
315/* Interactive316 ========================================================================== */317
318/*319 * Add the correct display in Edge, IE 10+, and Firefox.320 */321
322details {323 display: block;324}325
326/*327 * Add the correct display in all browsers.328 */329
330summary {331 display: list-item;332}333
334/* Misc335 ========================================================================== */336
337/**338 * Add the correct display in IE 10+.339 */340
341template {342 display: none;343}344
345/**346 * Add the correct display in IE 10.347 */348
349[hidden] {350 display: none;351}
Step 8: Create the main.css
file
To create the main.css
file, run the following command:
Click to expand the code of the `main.css` file
1* {2 box-sizing: border-box;3 padding: 0;4 margin: 0;5}6
7html {8 scroll-behavior: smooth;9 font-size: 62.5%;10}11
12body {13 font-family: "Roboto", sans-serif;14 font-size: 1.6rem;15 line-height: 1.6;16 color: #333;17}18
19header {20 display: flex;21 justify-content: space-around;22 align-items: center;23 background-color: #333;24 color: #fff;25 height: 10rem;26}27
28header > nav {29 display: flex;30 justify-content: space-around;31 align-items: center;32 width: 50%;33}34
35header > nav > ul {36 display: flex;37 justify-content: space-around;38 align-items: center;39 width: 100%;40}41
42header > nav > ul > li {43 list-style: none;44}45
46header > nav > ul > li > a {47 text-decoration: none;48 color: #fff;49}50
51main {52 display: flex;53 justify-content: center;54 align-items: center;55 flex-direction: column;56 height: 70vh;57}58
59main > h1 {60 font-size: 5rem;61 margin-bottom: 2rem;62}63
64main > p {65 font-size: 2rem;66 margin-bottom: 2rem;67}68
69footer {70 display: flex;71 justify-content: center;72 align-items: center;73 height: 10rem;74 background-color: #333;75 color: #fff;76}
Step 8: Add the files to the GitHub repository
To add the files to the GitHub repository, run the following command:
# Add the files to the GitHub repositorygit add .
# Commit the files to the GitHub repositorygit commit -m "build: add the files to the GitHub repository"
# Push the files to the GitHub repositorygit push origin devlopment
Create a Jenkins Pipeline
Step 1: Create a new Jenkins pipeline
To create a new Jenkins pipeline, run the following command:
# Create a new Jenkins pipelinetouch Jenkinsfile
Step 2: Add the code to the Jenkins pipeline
To add the code to the Jenkins pipeline, run the following command:
1pipeline {2 agent any3 environment {4 AWS_PUBLIC_IP_DEV = 'AWS_PUBLIC_IP_DEV'5 }6
7 stages {8 stage('Deploy to Development') {9 steps {10 sh '''11 # remove the files12 ssh -i "/var/lib/jenkins/.ssh/aws-key-pair.pem" -o StrictHostKeyChecking=no ec2-user@${AWS_PUBLIC_IP_DEV} "sudo rm -rf /var/www/html/*"13 # copy the files to the dev server14 scp -i "/var/lib/jenkins/.ssh/aws-key-pair.pem" -o StrictHostKeyChecking=no -r ./* ec2-user@${AWS_PUBLIC_IP_DEV}:/var/www/html15 # restart the apache server16 ssh -i "/var/lib/jenkins/.ssh/aws-key-pair.pem" -o StrictHostKeyChecking=no ec2-user@${AWS_PUBLIC_IP_DEV} "sudo systemctl restart httpd"17 '''18 }19 }20 }21}
Step 3: Add the files to the GitHub repository
To add the files to the GitHub repository, run the following command:
# Add the files to the GitHub repositorygit add .
# Commit the files to the GitHub repositorygit commit -m "build: add the jenkins pipeline to the GitHub repository"
# Push the files to the GitHub repositorygit push origin devlopment
Create a Jenkins Job
Step 1: Create a new Jenkins job
- Open the Jenkins dashboard at EC2 instance from previous blog post. Go to
http://<public-ip-address>:8080/
and login with the credentials you created in the previous blog post.
- Click on
New Item
and enter the name of the job. SelectPipeline
and click onOK
.
- Name:
Quick Test Jenkins Devlopment
- Select:
Pipeline
- Click on:
OK
- Configure the job.
- Description:
Quick Test Jenkins Devlopment
- Select:
Pipeline
- Definition:
Pipeline script from SCM
- SCM:
Git
- Repository URL:
https://github.com/MKAbuMattar/quick-test-jenkins.git
- Credentials:
Add
>Jenkins
>Global credentials (unrestricted)
>Add Credentials
>Username with password
>Kind: Username with password
>Username: <your-github-username>
>Password: <your-github-password>
>ID: <your-github-username>
>Description: <your-github-username>
>OK
>OK
>OK
- Branches to build:
*/devlopment
- Repository URL:
- Definition:
- Click on:
Save
Note: How to Add Git Credentials in Jenkins for private repositories on GitHub
Step 2: Build the Jenkins job
Before building the Jenkins job, you need to add the key pair to the Jenkins server.
- Connect to the Jenkins server using SSH.
# Connect to the Jenkins server using SSHssh -i "<path-to-key-pair>" ec2-user@<public-ip-address>
- Login to the Jenkins server.
# Login to the Jenkins serversudo -su jenkins
- Create a
.ssh
directory.
# Create a .ssh directorymkdir .ssh
# Change the directorycd .ssh
- Create a
aws-key-pair.pem
file.
# Create a aws-key-pair.pem filecat > aws-key-pair.pem << EOF-----BEGIN RSA PRIVATE KEY-----copy the private key from the key pair-----END RSA PRIVATE KEY-----EOF
- Change the permission of the
aws-key-pair.pem
file.
# Change the permission of the aws-key-pair.pem filechmod 400 aws-key-pair.pem
- Exit the Jenkins server.
# Exit the Jenkins serverexit
- Click on
Build Now
to build the Jenkins job.
- Click on
Console Output
to see the build logs.
Step 3: Build the Jenkins job Automatically on GitHub push
- Go to
GitHub
> go toquick-test-jenkins
repository- Click on
Settings
- Click on
Webhooks
- Click on
Add webhook
- Payload URL:
http://<public-ip-address>:8080/github-webhook/
- Content type:
application/x-www-form-urlencoded
- Which events would you like to trigger this webhook?:
Just the push event.
- Active:
Yes
- Click on:
Add webhook
- Payload URL:
- Click on
- Click on
- Click on
- Go to
Jenkins
>Quick Test Jenkins Devlopment
job- Click on
Configure
- Select:
GitHub hook trigger for GITScm polling
- Click on:
Save
- Select:
- Click on
Step 4: Test the Jenkins job Automatically on GitHub push
We’ll add new files to the devlopment
branch and push them to the GitHub repository.
1<!DOCTYPE html>2<html>3 <head>4 <meta charset="utf-8" />5 <meta name="viewport" content="width=device-width, initial-scale=1" />6 <title><?php echo ENV; ?> | My Site</title>7 <link rel="stylesheet" href="./assets/css/normalize.css" />8 <link rel="stylesheet" href="./assets/css/main.css" />9 </head>10 <body>11 <header>12 <h1>My Site</h1>13
14 <nav>15 <ul>16 <li><a href="./index.php">Home</a></li>17 <li><a href="./table.php">Table</a></li>18 </ul>19 </nav>20 </header>21
22 <main>23 <h2>Home</h2>24 <p>Welcome to my site!</p>25 <p>26 <?php echo "Environment: " . ENV; ?>27 </p>28
29 <table border="1">30 <thead>31 <tr>32 <th>ID</th>33 <th>Name</th>34 <th>Email</th>35 </tr>36 </thead>37 <tbody>38 <?php foreach ($users as $user) : ?>39 <tr>40 <td><?php echo $user['id']; ?></td>41 <td><?php echo $user['name']; ?></td>42 <td><?php echo $user['email']; ?></td>43 </tr>44 <?php endforeach; ?>45 </tbody>46 </table>47 </main>48
49 <footer>50 <p>My Site © 2022</p>51 </footer>52 </body>53</html>
1<!DOCTYPE html>2<html>3 <head>4 <meta charset="utf-8" />5 <meta name="viewport" content="width=device-width, initial-scale=1" />6 <title><?php echo ENV; ?> | My Site</title>7 <link rel="stylesheet" href="./assets/css/normalize.css" />8 <link rel="stylesheet" href="./assets/css/main.css" />9 </head>10 <body>11 <header>12 <h1>My Site</h1>13
14 <nav>15 <ul>16 <li><a href="./index.php">Home</a></li>17 <li><a href="./table.php">Table</a></li>18 </ul>19 </nav>20 </header>21
22 <main>23 <h2>Home</h2>24 <p>Welcome to my site!</p>25 <p>26 <?php echo "Environment: " . ENV; ?>27 </p>28 </main>29
30 <footer>31 <p>My Site © 2022</p>32 </footer>33 </body>34</html>
1<?php2
3// Include the controller file4require_once 'app/controllers/index.php';5
6// Include the view file7require_once 'app/views/tabs.php';
Before we push the files to the GitHub repository, we’ll open the EC2 instance in the browser to see the changes.
# Add the files to the GitHub repositorygit add .
# Commit the files to the GitHub repositorygit commit -m "build: test the Jenkins job Automatically on GitHub push"
# Push the files to the GitHub repositorygit push origin devlopment
- Go to
Jenkins
>Quick Test Jenkins Devlopment
job >Build History
.
- Click on
Build #2
to see the build details.
- Open the EC2 instance in the browser to see the changes.
Step 5: We’ll do the Same Changes for Jenkinsfile
file
# create a new directorymkdir -p .build
# create a new directory for development and productionmkdir -p .build/dev .build/prod
# move the Jenkinsfile file to the development directorymv Jenkinsfile .build/dev
# create a new Jenkinsfile file for productiontouch .build/prod/Jenkinsfile
1pipeline {2 agent any3 environment {4 AWS_PUBLIC_IP_PROD = ''5 }6
7 stages {8 stage('Deploy to Production') {9 steps {10 sh '''11 # remove the files12 ssh -i "/var/lib/jenkins/.ssh/aws-key-pair.pem" -o StrictHostKeyChecking=no ec2-user@${AWS_PUBLIC_IP_PROD} "sudo rm -rf /var/www/html/*"13 # copy the files to the prod server14 scp -i "/var/lib/jenkins/.ssh/aws-key-pair.pem" -o StrictHostKeyChecking=no -r ./* ec2-user@${AWS_PUBLIC_IP_PROD}:/var/www/html15 # restart the apache server16 ssh -i "/var/lib/jenkins/.ssh/aws-key-pair.pem" -o StrictHostKeyChecking=no ec2-user@${AWS_PUBLIC_IP_PROD} "sudo systemctl restart httpd"17 '''18 }19 }20 }21}
Step 6: Do Some Changes at Jenkins Configure for Development Jenkins Job
- Go to
Jenkins
>Quick Test Jenkins Devlopment
job >Configure
. - Scroll down to
Script Path
and change the value toJenkinsfile
to.build/dev/Jenkinsfile
.
Step 7: Setup the Production Jenkins job
the Production Jenkins job will be triggered automatically when the Development Jenkins job is successful merge to the main
branch.
- Click on
New Item
and enter the name of the job. SelectPipeline
and click onOK
.
- Name:
Quick Test Jenkins Production
- Select:
Pipeline
- Click on:
OK
- Configure the job.
- Description:
Quick Test Jenkins Production
- Select:
Poll SCM
- Schedule:
H/5 * * * *
- Select:
Ignore post-commit hooks
- Schedule:
- Select:
Pipeline
- Definition:
Pipeline script from SCM
- SCM:
Git
- Repository URL:
https://github.com/MKAbuMattar/quick-test-jenkins.git
- Credentials:
Add
>Jenkins
>Global credentials (unrestricted)
>Add Credentials
>Username with password
>Kind: Username with password
>Username: <your-github-username>
>Password: <your-github-password>
>ID: <your-github-username>
>Description: <your-github-username>
>OK
>OK
>OK
- Branches to build:
*/main
- Repository URL:
- Definition:
- Click on:
Save
Step 8: Go to GitHub and Merge the devlopment
branch to the main
branch
- Go to
GitHub
>quick-test-jenkins
repository >devlopment
branch >Pull requests
>New pull request
.
Check the EC2 instance in the browser to see the changes, and you’ll see the changes.
Conclusion
In this tutorial, we learned how to setup a CI/CD pipeline using Jenkins and GitHub. We also learned how to setup a Jenkins job to automatically deploy the code to the AWS EC2 instance.